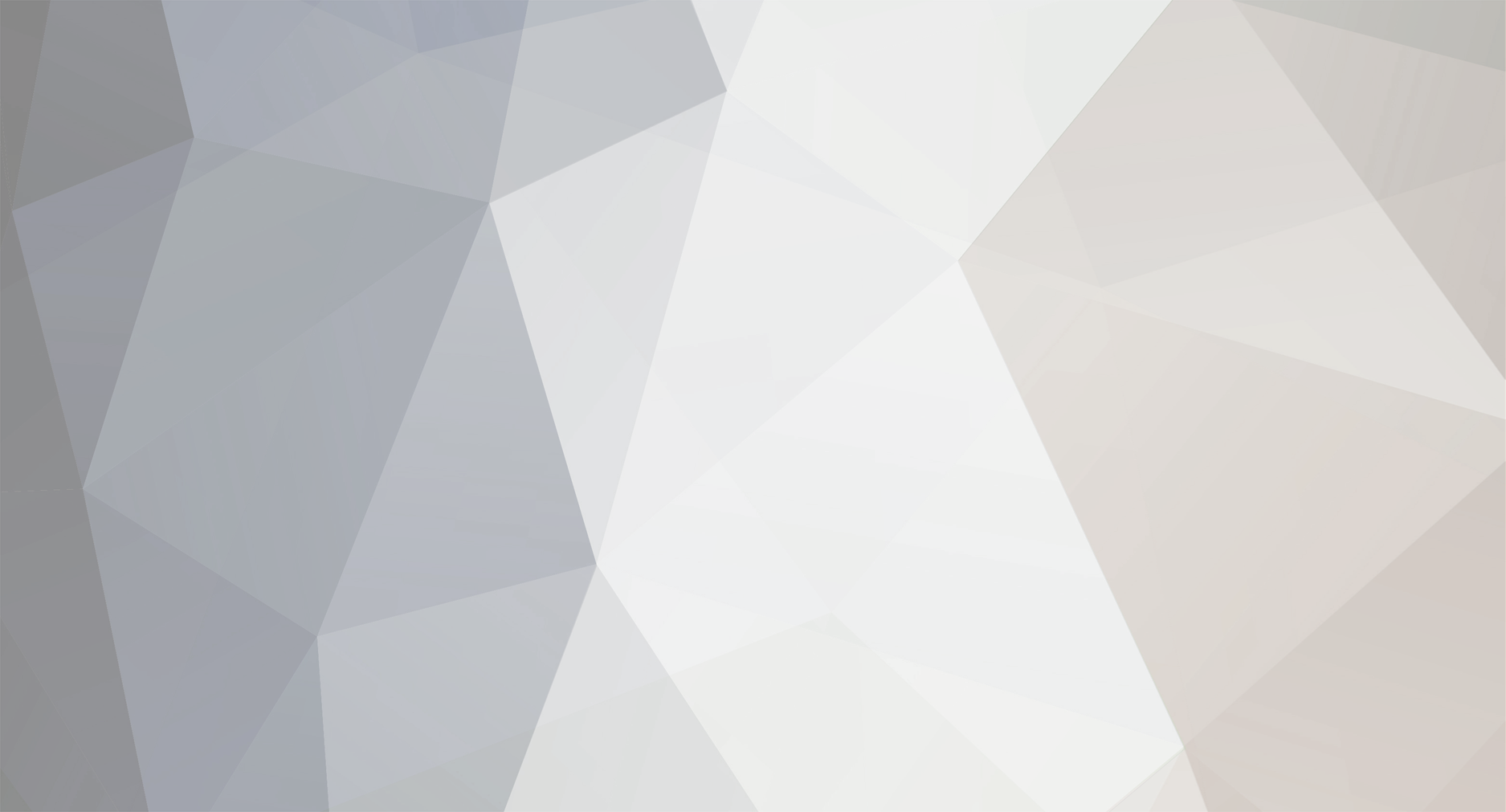
shadowmasteur
Members-
Posts
7 -
Credits
0 -
Joined
-
Last visited
-
Feedback
0%
Content Type
Articles
Profiles
Forums
Store
Everything posted by shadowmasteur
-
Help The quest does not show up in the quests menu
shadowmasteur replied to shadowmasteur's question in Request Server Development Help [L2J]
i modified /* * Copyright © 2004-2021 L2J DataPack * * This file is part of L2J DataPack. * * L2J DataPack is free software: you can redistribute it and/or modify * it under the terms of the GNU General Public License as published by * the Free Software Foundation, either version 3 of the License, or * (at your option) any later version. * * L2J DataPack is distributed in the hope that it will be useful, * but WITHOUT ANY WARRANTY; without even the implied warranty of * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU * General Public License for more details. * * You should have received a copy of the GNU General Public License * along with this program. If not, see <http://www.gnu.org/licenses/>. */ package com.l2jserver.datapack.handlers.bypasshandlers; import java.util.ArrayList; import java.util.HashSet; import java.util.List; import java.util.Set; import java.util.logging.Level; import com.l2jserver.gameserver.data.xml.impl.NpcData; import com.l2jserver.gameserver.handler.IBypassHandler; import com.l2jserver.gameserver.instancemanager.QuestManager; import com.l2jserver.gameserver.model.L2World; import com.l2jserver.gameserver.model.actor.L2Character; import com.l2jserver.gameserver.model.actor.L2Npc; import com.l2jserver.gameserver.model.actor.instance.L2PcInstance; import com.l2jserver.gameserver.model.actor.templates.L2NpcTemplate; import com.l2jserver.gameserver.model.events.EventType; import com.l2jserver.gameserver.model.events.listeners.AbstractEventListener; import com.l2jserver.gameserver.model.quest.Quest; import com.l2jserver.gameserver.model.quest.QuestState; import com.l2jserver.gameserver.network.SystemMessageId; import com.l2jserver.gameserver.network.serverpackets.ActionFailed; import com.l2jserver.gameserver.network.serverpackets.NpcHtmlMessage; import com.l2jserver.gameserver.util.StringUtil; public class QuestLink implements IBypassHandler { private static final int MAX_QUEST_COUNT = 40; private static final int TO_LEAD_AND_BE_LED = 118; private static final int THE_LEADER_AND_THE_FOLLOWER = 123; private static final String[] COMMANDS = { "Quest" }; @Override public boolean useBypass(String command, L2PcInstance activeChar, L2Character target) { String quest = ""; try { quest = command.substring(5).trim(); } catch (IndexOutOfBoundsException ioobe) { } if (quest.length() == 0) { showQuestWindow(activeChar, (L2Npc) target); } else { int questNameEnd = quest.indexOf(" "); if (questNameEnd == -1) { showQuestWindow(activeChar, (L2Npc) target, quest); } else { activeChar.processQuestEvent(quest.substring(0, questNameEnd), quest.substring(questNameEnd).trim()); } } return true; } /** * Open a choose quest window on client with all quests available of the L2NpcInstance.<br> * <b><u>Actions</u>:</b><br> * <li>Send a Server->Client NpcHtmlMessage containing the text of the L2NpcInstance to the L2PcInstance</li> * @param player The L2PcInstance that talk with the L2NpcInstance * @param npc The table containing quests of the L2NpcInstance * @param quests the quest available */ private static void showQuestChooseWindow(L2PcInstance player, L2Npc npc, Quest[] quests) { final StringBuilder sb = StringUtil.startAppend(150, "<html><body>"); String state = ""; String color = ""; int questId = -1; for (Quest quest : quests) { if (quest == null) { continue; } final QuestState qs = player.getQuestState(quest.getName()); if ((qs == null) || qs.isCreated()) { state = quest.isCustomQuest() ? "" : "01"; if (quest.canStartQuest(player)) { color = "bbaa88"; } else { color = "a62f31"; } } else if (qs.isStarted()) { state = quest.isCustomQuest() ? " (In Progress)" : "02"; color = "ffdd66"; } else if (qs.isCompleted()) { state = quest.isCustomQuest() ? " (Done)" : "03"; color = "787878"; } StringUtil.append(sb, "<a action=\"bypass -h npc_", String.valueOf(npc.getObjectId()), "_Quest ", quest.getName(), "\">"); StringUtil.append(sb, "<font color=\"" + color + "\">["); if (quest.isCustomQuest()) { StringUtil.append(sb, quest.getDescr(), state); } else { questId = quest.getId(); if (questId > 80000) { questId -= 5000; } else if (questId == 146) { questId = 640; } StringUtil.append(sb, "<fstring>", String.valueOf(questId), state, "</fstring>"); } sb.append("]</font></a><br>"); if ((player.getApprentice() > 0) && (L2World.getInstance().getPlayer(player.getApprentice()) != null)) { if (questId == TO_LEAD_AND_BE_LED) { sb.append("<a action=\"bypass -h Quest Q00118_ToLeadAndBeLed sponsor\"><font color=\"").append(color) // .append("\">[<fstring>").append(questId).append(state).append("</fstring> (Sponsor)]</font></a><br>"); } if (questId == THE_LEADER_AND_THE_FOLLOWER) { sb.append("<a action=\"bypass -h Quest Q00123_TheLeaderAndTheFollower sponsor\"><font color=\"").append(color) // .append("\">[<fstring>").append(questId).append(state).append("</fstring> (Sponsor)]</font></a><br>"); } } } sb.append("</body></html>"); // Send a Server->Client packet NpcHtmlMessage to the L2PcInstance in order to display the message of the L2NpcInstance npc.insertObjectIdAndShowChatWindow(player, sb.toString()); } /** * Open a quest window on client with the text of the L2NpcInstance.<br> * <b><u>Actions</u>:</b><br> * <ul> * <li>Get the text of the quest state in the folder com/l2jserver/datapack/quests/questId/stateId.htm</li> * <li>Send a Server->Client NpcHtmlMessage containing the text of the L2NpcInstance to the L2PcInstance</li> * <li>Send a Server->Client ActionFailed to the L2PcInstance in order to avoid that the client wait another packet</li> * </ul> * @param player the L2PcInstance that talk with the {@code npc} * @param npc the L2NpcInstance that chats with the {@code player} * @param questId the Id of the quest to display the message */ private static void showQuestWindow(L2PcInstance player, L2Npc npc, String questId) { String content = null; final Quest q = QuestManager.getInstance().getQuest(questId); // Get the state of the selected quest final QuestState qs = player.getQuestState(questId); if (q != null) { if (((q.getId() >= 1) && (q.getId() < 80000)) && ((player.getWeightPenalty() >= 3) || !player.isInventoryUnder90(true))) { player.sendPacket(SystemMessageId.INVENTORY_LESS_THAN_80_PERCENT); return; } if (qs == null) { if ((q.getId() >= 1) && (q.getId() < 80000)) { // Too many ongoing quests. if (player.getAllActiveQuests().size() >= MAX_QUEST_COUNT) { final NpcHtmlMessage html = new NpcHtmlMessage(npc.getObjectId()); html.setFile(player.getHtmlPrefix(), "data/html/fullquest.html"); player.sendPacket(html); return; } } } q.notifyTalk(npc, player); } else { content = Quest.getNoQuestMsg(player); // no quests found } // Send a Server->Client packet NpcHtmlMessage to the L2PcInstance in order to display the message of the L2NpcInstance if (content != null) { npc.insertObjectIdAndShowChatWindow(player, content); } // Send a Server->Client ActionFailed to the L2PcInstance in order to avoid that the client wait another packet player.sendPacket(ActionFailed.STATIC_PACKET); } /** * @param player the player talking to the NPC * @param npcId The Identifier of the NPC * @return a table containing all QuestState from the table _quests in which the L2PcInstance must talk to the NPC. */ private static List<QuestState> getQuestsForTalk(L2PcInstance player, int npcId) { // Create a QuestState table that will contain all QuestState to modify final List<QuestState> states = new ArrayList<>(); final L2NpcTemplate template = NpcData.getInstance().getTemplate(npcId); if (template == null) { _log.log(Level.WARNING, QuestLink.class.getSimpleName() + ": " + player.getName() + " requested quests for talk on non existing npc " + npcId); return states; } // Go through the QuestState of the L2PcInstance quests for (AbstractEventListener listener : template.getListeners(EventType.ON_NPC_TALK)) { if (listener.getOwner() instanceof Quest) { final Quest quest = (Quest) listener.getOwner(); if (quest.isVisibleInQuestWindow()) { // Copy the current L2PcInstance QuestState in the QuestState table final QuestState st = player.getQuestState(quest.getName()); if (st != null) { states.add(st); } } } } // Return a table containing all QuestState to modify return states; } /** * Collect awaiting quests/start points and display a QuestChooseWindow (if several available) or QuestWindow. * @param player the L2PcInstance that talk with the {@code npc}. * @param npc the L2NpcInstance that chats with the {@code player}. */ public static void showQuestWindow(L2PcInstance player, L2Npc npc) { boolean conditionMeet = false; final Set<Quest> options = new HashSet<>(); for (QuestState state : getQuestsForTalk(player, npc.getId())) { final Quest quest = state.getQuest(); if (quest == null) { _log.log(Level.WARNING, player + " Requested incorrect quest state for non existing quest: " + state.getQuestName()); continue; } if ((quest.getId() > 0) && (quest.getId() < 80000)) { options.add(quest); if (quest.canStartQuest(player)) { conditionMeet = true; } } } for (AbstractEventListener listener : npc.getListeners(EventType.ON_NPC_QUEST_START)) { if (listener.getOwner() instanceof Quest) { final Quest quest = (Quest) listener.getOwner(); if (quest.isVisibleInQuestWindow()) { if ((quest.getId() > 0) && (quest.getId() < 80000)) { options.add(quest); if (quest.canStartQuest(player)) { conditionMeet = true; } } } } } if (!conditionMeet) { showQuestWindow(player, npc, ""); } else if ((options.size() > 1) || ((player.getApprentice() > 0) && (L2World.getInstance().getPlayer(player.getApprentice()) != null) && options.stream().anyMatch(q -> q.getId() == TO_LEAD_AND_BE_LED))) { showQuestChooseWindow(player, npc, options.toArray(new Quest[options.size()])); } else if (options.size() == 1) { showQuestWindow(player, npc, options.stream().findFirst().get().getName()); } else { showQuestWindow(player, npc, ""); } } @Override public String[] getBypassList() { return COMMANDS; } } ID >20000 is not a problem now -
Help The quest does not show up in the quests menu
shadowmasteur replied to shadowmasteur's question in Request Server Development Help [L2J]
Hello, I use Lineage 2 High Five. -
Hello everyone ! I created a homemade quest it works very well but it does not appear in the quests menu I have however added cessis in questname-e.dat 1 40000 1 a,Test\0 a,Livret l'objet\0 a,TESTA vous demande d'apporter l'objet TEST à TESTB.\\n\0 0 0 -84108.00000000 244604.00000000 -3729.00000000 0 0 3 a,Test Quête B\0 1 1 1 30048 -84436.00000000 242793.00000000 -3729.00000000 a,No Requirements\0 a,Livret l'objet a TESTB.\0 0 0 0 0 0 30 0 4 15623 15624 57 906 4 5672 446 2466 1 1 0 1 40000 4294967295 a,Jewel of Valakas\0 a, a, 0 0 183664.00000000 -114944.00000000 -3335.00000000 84 0 1 a, 1 1 1 31540 183664.00000000 -114944.00000000 -3335.00000000 a,No record of having completed this quest today\0 a,There is a mysterious jewel that teleports you very close to the Hall of Flames.\0 0 1 7267 0 0 0 187 0 1 21896 1 1 1 2 If you could give me a line of research because I have no idea of the problem thank you in advance good evening / day to you
-
Request Item invisible...
shadowmasteur replied to shadowmasteur's topic in Request Support [English]
I finally succeeded... I'm an asshole... in fact I have several installations of the Lineage II game on the PC and I don't modify the one I launch... I'm sorry to have made you lose your time -_-' -
Request Item invisible...
shadowmasteur replied to shadowmasteur's topic in Request Support [English]
Hello, sorry, google chrome has translated the [English] section into [French] for some reason, so I got tricked... I have a problem I configured an object in order to create a new one here is what I did: in l2jserver\server\Game\data\stats\items\40000-40100.xml <?xml version="1.0" encoding="UTF-8"?> <list xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="../../../xsd/items.xsd"> <item id="40000" type="EtcItem" name="test"> <!-- Premier objet pour testé une quête réplique du 17238 --> <set name="icon" val="icon.mercenary_certification_i00" /> <set name="material" val="PAPER" /> <set name="is_tradable" val="false" /> <set name="is_droppable" val="false" /> <set name="is_sellable" val="false" /> <set name="is_depositable" val="false" /> <set name="is_stackable" val="true" /> <set name="is_questitem" val="true" /> </item> </list> I modified the files: (the new one is 40,000 the original model is 17,238) etcitemgrp.dat 17238 Certificat de participation au match 3 de l'Olympiade a,Document prouvant la participation au match 3 de l'Olympiade. Apportez ce document à l'opérateur de l'Olympiade pour recevoir la récompense minimale.\0 -1 0 0 a, 0 0 a, 0 0 0 0 0 0 0 0 0 0 un, 1 40000 Certificat de participation au Match 3 de l'Olympiade a,Document prouvant la participation au Match 3 de l'Olympiade. Apportez ce document à l'Opérateur de l'Olympiade pour recevoir la récompense minimale.\0 -1 0 0 a, 0 0 a, 0 0 0 0 0 0 0 0 0 0 un, 1 itemname-e.dat 17238 Certificat de participation au match 3 de l'Olympiade a,Document prouvant la participation au match 3 de l'Olympiade. Apportez ce document à l'opérateur de l'Olympiade pour recevoir la récompense minimale.\0 -1 0 0 a, 0 0 a, 0 0 0 0 0 0 0 0 0 0 un, 1 40000 Certificat de participation au Match 3 de l'Olympiade a,Document prouvant la participation au Match 3 de l'Olympiade. Apportez ce document à l'Opérateur de l'Olympiade pour recevoir la récompense minimale.\0 -1 0 0 a, 0 0 a, 0 0 0 0 0 0 0 0 0 0 un, 1 I left the same names because the item does not appear in the game. in the database my character has the item... but only the object 17 238 appears the other remains invisible I don't know what to do anymore Could you help me ? I shared the file: https://mega.nz/file/dVglXBjD#H60X16LIrd4Ry-COk3CjjyAyB4yoI3qrQdggJhQpr2Q here are the two files: itemname-e.dat and etcitemgrp.dat Thanks in advance ! -
Bonjour à tous j'ai un soucis j'ai configuré un objet afin d'en créer un nouveau voila ce que j'ai fais : dans l2jserver\server\Game\data\stats\items\40000-40100.xml < ? xml version = "1.0" encoding = "UTF-8" ?> <list xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation = "../../. ./xsd/items.xsd" > <item id = "40000" type = "EtcItem" name = "test" > <!-- Premier objet pour testé une quête réplique du 17238 --> <set name = "icon" val = "icône. "PAPIER" /> < nom de l'ensemble = "is_tradable" val = "false" /> < nom de l'ensemble = "is_droppable" val = "false" /> < nom de l'ensemble = "is_sellable" val = "false" /> < nom de l'ensemble = "is_depositable" val = "false" /> <set name = "is_stackable" val = "true"/> <set name = "is_questitem"val = "vrai" /> </item> </list> j'ai modifier les fichiers : (le nouveau est le 40 000 le modèle original est le 17 238) etcitemgrp.dat 2 17238 0 3 0 0 0 0 0 0 0 0 0 0 0 icon.mercenary_certification_i00 -1 0 18 0 0 0 1 1 1 [aucun] [aucun] 0 0 0 2 40000 0 3 0 0 0 0 0 0 0 0 0 0 0 icon.mercenary_certification_i00 -1 0 18 0 0 0 1 1 1 [aucun] [aucun] 0 0 0 itemname-e.dat 17238 Certificat de participation au match 3 de l'Olympiade a,Document prouvant la participation au match 3 de l'Olympiade. Apportez ce document à l'opérateur de l'Olympiade pour recevoir la récompense minimale.\0 -1 0 0 a, 0 0 a, 0 0 0 0 0 0 0 0 0 0 un, 1 40000 Certificat de participation au Match 3 de l'Olympiade a,Document prouvant la participation au Match 3 de l'Olympiade. Apportez ce document à l'Opérateur de l'Olympiade pour recevoir la récompense minimale.\0 -1 0 0 a, 0 0 a, 0 0 0 0 0 0 0 0 0 0 un, 1 j'ai laissé les mêmes noms car l'item n'apparaît pas dans le jeu. dans la base de données mon personnage possède bien l'item... mais seul l'objet 17 238 apparait l'autre reste invisible je ne c'est plus quoi faire Pourriez-vous m'aider ? j'ai partagé le fichier : https://mega.nz/file/dVglXBjD#H60X16LIrd4Ry-COk3CjjyAyB4yoI3qrQdggJhQpr2Q il y a les deux fichiers : itemname-e.dat et etcitemgrp.dat Merci d'avance !