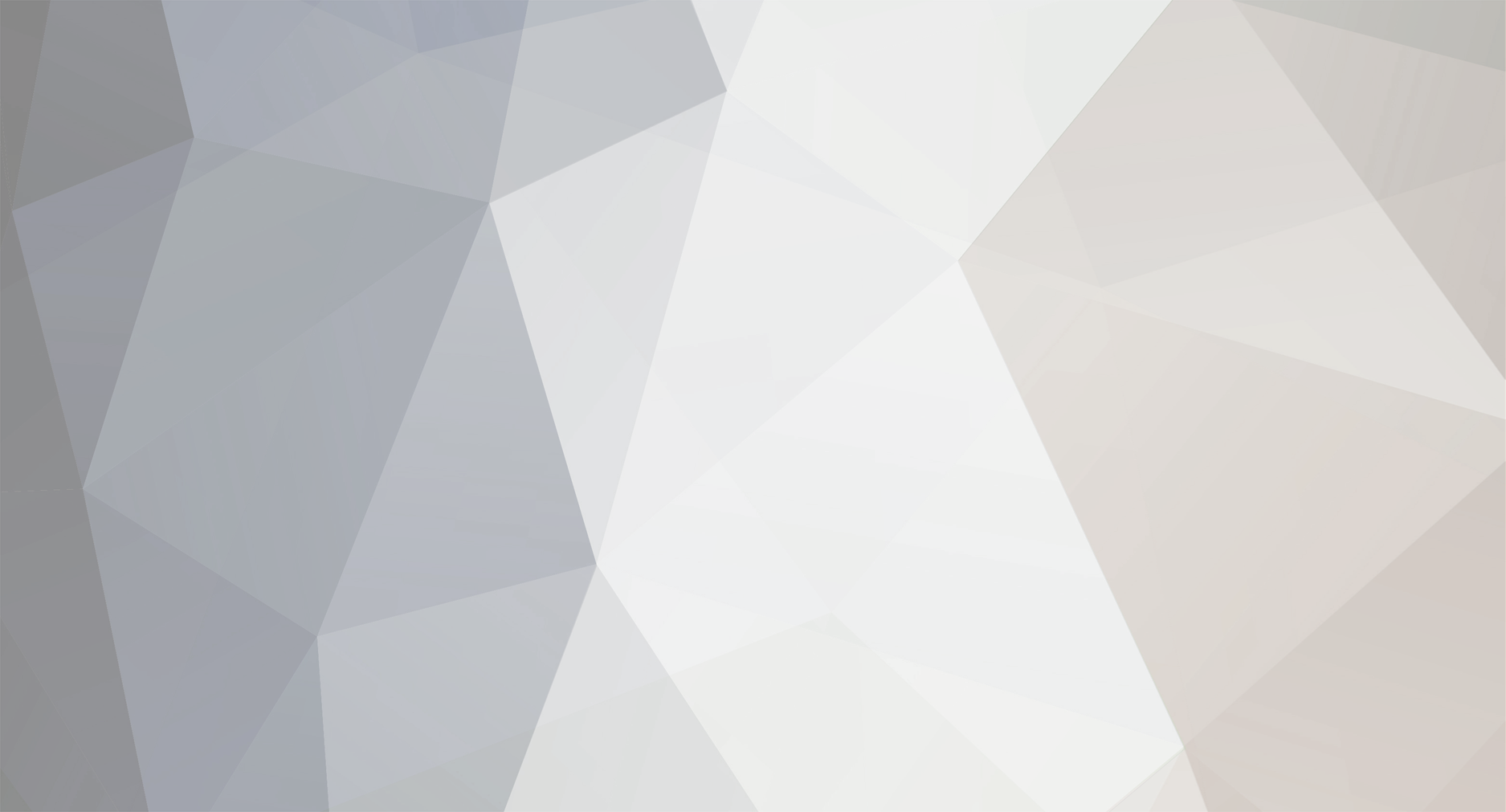
streamss
Members-
Posts
30 -
Credits
0 -
Joined
-
Last visited
Never -
Feedback
0%
Content Type
Articles
Profiles
Forums
Store
Everything posted by streamss
-
it's player in client? or playmusiic 1? dll of java diff?
-
for thank have karma. add+1
-
reupload plz
-
[UPDATED-Share]New event - Biohazard
streamss replied to azizilaika's topic in Server Shares & Files [L2J]
/* * This program is free software: you can redistribute it and/or modify it under * the terms of the GNU General Public License as published by the Free Software * Foundation, either version 3 of the License, or (at your option) any later * version. * * This program is distributed in the hope that it will be useful, but WITHOUT * ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS * FOR A PARTICULAR PURPOSE. See the GNU General Public License for more * details. * * You should have received a copy of the GNU General Public License along with * this program. If not, see <http://www.gnu.org/licenses/>. */ package handlers.voicedcommandhandlers; import net.sf.l2j.gameserver.handler.IVoicedCommandHandler; import net.sf.l2j.gameserver.instancemanager.CastleManager; import net.sf.l2j.gameserver.model.actor.instance.L2DoorInstance; import net.sf.l2j.gameserver.model.actor.instance.L2PcInstance; import net.sf.l2j.gameserver.model.entity.Castle; import net.sf.l2j.gameserver.model.entity.Biohazard; /** * * */ public class castle implements IVoicedCommandHandler { private static final String[] VOICED_COMMANDS = { "open doors", "close doors", "ride wyvern", "bhreg", "bhunreg" }; /** * * @see net.sf.l2j.gameserver.handler.IVoicedCommandHandler#useVoicedCommand(java.lang.String, net.sf.l2j.gameserver.model.actor.instance.L2PcInstance, java.lang.String) */ public boolean useVoicedCommand(String command, L2PcInstance activeChar, String target) { if (command.startsWith("open doors") && target.equals("castle") && (activeChar.isClanLeader())) { L2DoorInstance door = (L2DoorInstance) activeChar.getTarget(); Castle castle = CastleManager.getInstance().getCastleById(activeChar.getClan().getHasCastle()); if (door == null || castle == null) return false; if (castle.checkIfInZone(door.getX(), door.getY(), door.getZ())) { door.openMe(); } } else if (command.startsWith("close doors") && target.equals("castle") && (activeChar.isClanLeader())) { L2DoorInstance door = (L2DoorInstance) activeChar.getTarget(); Castle castle = CastleManager.getInstance().getCastleById(activeChar.getClan().getHasCastle()); if (door == null || castle == null) return false; if (castle.checkIfInZone(door.getX(), door.getY(), door.getZ())) { door.closeMe(); } } else if (command.startsWith("ride wyvern") && target.equals("castle")) { if (activeChar.getClan().getHasCastle() > 0 && activeChar.isClanLeader()) { activeChar.mount(12621, 0, true); } } else if (command.equalsIgnoreCase("bhreg")) Biohazard.addParticipant(activeChar); else if (command.equalsIgnoreCase("bhunreg")) Biohazard.removeParticipant(activeChar); return true; } /** * * @see net.sf.l2j.gameserver.handler.IVoicedCommandHandler#getVoicedCommandList() */ public String[] getVoicedCommandList() { return VOICED_COMMANDS; } } -
TheFostOK this event don't work correctly.
-
[UPDATED-Share]New event - Biohazard
streamss replied to azizilaika's topic in Server Shares & Files [L2J]
jaden latest code test on gracia final -
patch works fine, but i added here also the removal of expired "bans", because without it, the IP could not login till gameserver restart (bad for LAN house..) Index: java/com/l2jserver/gameserver/GameServer.java =================================================================== --- java/com/l2jserver/gameserver/GameServer.java (revision 3703) +++ java/com/l2jserver/gameserver/GameServer.java (working copy) @@ -437,7 +437,7 @@ sc.HELPER_BUFFER_COUNT = Config.MMO_HELPER_BUFFER_COUNT; final L2GamePacketHandler gph = new L2GamePacketHandler(); - _selectorThread = new SelectorThread<L2GameClient>(sc, gph, gph, gph, null); + _selectorThread = new SelectorThread<L2GameClient>(sc, gph, gph, gph, gph); InetAddress bindAddress = null; if (!Config.GAMESERVER_HOSTNAME.equals("*")) Index: java/com/l2jserver/gameserver/network/L2GamePacketHandler.java =================================================================== --- java/com/l2jserver/gameserver/network/L2GamePacketHandler.java (revision 3703) +++ java/com/l2jserver/gameserver/network/L2GamePacketHandler.java (working copy) @@ -14,11 +14,17 @@ */ package com.l2jserver.gameserver.network; +import java.net.InetAddress; import java.nio.ByteBuffer; +import java.nio.channels.SocketChannel; +import java.util.ArrayList; +import java.util.HashMap; +import java.util.Map.Entry; import java.util.concurrent.RejectedExecutionException; import java.util.logging.Logger; +import org.mmocore.network.IAcceptFilter; import org.mmocore.network.IClientFactory; import org.mmocore.network.IMMOExecutor; import org.mmocore.network.IPacketHandler; @@ -29,6 +35,8 @@ import com.l2jserver.gameserver.ThreadPoolManager; import com.l2jserver.gameserver.network.L2GameClient.GameClientState; import com.l2jserver.gameserver.network.clientpackets.*; +import com.l2jserver.loginserver.SelectorHelper; +import com.l2jserver.loginserver.SelectorHelper.Flood; import com.l2jserver.util.Util; /** @@ -40,9 +48,51 @@ * Note: If for a given exception a packet needs to be handled on more then one state, then it should be added to all these states. * @author KenM */ -public final class L2GamePacketHandler implements IPacketHandler<L2GameClient>, IClientFactory<L2GameClient>, IMMOExecutor<L2GameClient> +public final class L2GamePacketHandler implements IPacketHandler<L2GameClient>, IClientFactory<L2GameClient>, IMMOExecutor<L2GameClient>, IAcceptFilter { private static final Logger _log = Logger.getLogger(L2GamePacketHandler.class.getName()); + private final HashMap<Integer, Flood> _ipFloodMap = new HashMap<Integer, Flood>(); + + public L2GamePacketHandler() + { + super(); + Thread cleanUpIpFloodMap = new Thread() + { + public void run() + { + while (true) + { + long reference = System.currentTimeMillis() - (1000 * 300); + ArrayList<Integer> toRemove = new ArrayList<Integer>(); + synchronized (_ipFloodMap) + { + for (Entry<Integer, Flood> e : _ipFloodMap.entrySet()) + { + Flood f = e.getValue(); + if (f.lastAccess < reference) + toRemove.add(e.getKey()); + } + } + + synchronized (_ipFloodMap) + { + _ipFloodMap.keySet().removeAll(toRemove); + } + + try + { + Thread.sleep(5000); + } + catch (InterruptedException e) + { + + } + } + } + }; + cleanUpIpFloodMap.setDaemon(true); + cleanUpIpFloodMap.start(); + } // implementation public ReceivablePacket<L2GameClient> handlePacket(ByteBuffer buf, L2GameClient client) @@ -1130,4 +1180,52 @@ } } } + + @Override + public boolean accept(SocketChannel sc) + { + InetAddress addr = sc.socket().getInetAddress(); + int h = SelectorHelper.hash(addr.getAddress()); + + long current = System.currentTimeMillis(); + Flood f; + synchronized (_ipFloodMap) + { + f = _ipFloodMap.get(h); + } + if (f != null) + { + if (f.trys == -1) + { + f.lastAccess = current; + return false; + } + + if (f.lastAccess + 1000 > current) + { + f.lastAccess = current; + + if (f.trys >= 3) + { + f.trys = -1; + return false; + } + + f.trys++; + } + else + { + f.lastAccess = current; + } + } + else + { + synchronized (_ipFloodMap) + { + _ipFloodMap.put(h, new Flood()); + } + } + + return true; + } } Index: java/com/l2jserver/loginserver/SelectorHelper.java =================================================================== --- java/com/l2jserver/loginserver/SelectorHelper.java (revision 3703) +++ java/com/l2jserver/loginserver/SelectorHelper.java (working copy) @@ -34,8 +34,7 @@ * * @author KenM */ -public class SelectorHelper extends Thread implements IMMOExecutor<L2LoginClient>, - IClientFactory<L2LoginClient>, IAcceptFilter +public class SelectorHelper extends Thread implements IMMOExecutor<L2LoginClient>, IClientFactory<L2LoginClient>, IAcceptFilter { private HashMap<Integer, Flood> _ipFloodMap; private ThreadPoolExecutor _generalPacketsThreadPool; @@ -123,18 +122,17 @@ * @param ip * @return */ - private int hash(byte[] ip) + public static int hash(byte[] ip) { - return ip[0] & 0xFF | ip[1] << 8 & 0xFF00 | ip[2] << 16 & 0xFF0000 | ip[3] << 24 - & 0xFF000000; + return ip[0] & 0xFF | ip[1] << 8 & 0xFF00 | ip[2] << 16 & 0xFF0000 | ip[3] << 24 & 0xFF000000; } - private class Flood + public static class Flood { - long lastAccess; - int trys; + public long lastAccess; + public int trys; - Flood() + public Flood() { lastAccess = System.currentTimeMillis(); trys = 0; @@ -151,7 +149,7 @@ while (true) { long reference = System.currentTimeMillis() - (1000 * 300); - ArrayList<Integer> toRemove = new ArrayList<Integer>(50); + ArrayList<Integer> toRemove = new ArrayList<Integer>(); synchronized (_ipFloodMap) { for (Entry<Integer, Flood> e : _ipFloodMap.entrySet()) @@ -164,10 +162,7 @@ synchronized (_ipFloodMap) { - for (Integer i : toRemove) - { - _ipFloodMap.remove(i); - } + _ipFloodMap.keySet().removeAll(toRemove); } try [c]DrHouse and janiii
-
this as a temporary solution for L2j-Killer fix this, [c]DS Index: java/com/l2jserver/gameserver/GameServer.java =================================================================== --- java/com/l2jserver/gameserver/GameServer.java (revision 3728) +++ java/com/l2jserver/gameserver/GameServer.java (working copy) @@ -437,7 +437,7 @@ sc.HELPER_BUFFER_COUNT = Config.MMO_HELPER_BUFFER_COUNT; final L2GamePacketHandler gph = new L2GamePacketHandler(); - _selectorThread = new SelectorThread<L2GameClient>(sc, gph, gph, gph, null); + _selectorThread = new SelectorThread<L2GameClient>(sc, gph, gph, gph, gph); InetAddress bindAddress = null; if (!Config.GAMESERVER_HOSTNAME.equals("*")) Index: java/com/l2jserver/gameserver/network/L2GamePacketHandler.java =================================================================== --- java/com/l2jserver/gameserver/network/L2GamePacketHandler.java (revision 3728) +++ java/com/l2jserver/gameserver/network/L2GamePacketHandler.java (working copy) @@ -14,11 +14,15 @@ */ package com.l2jserver.gameserver.network; +import java.net.InetAddress; import java.nio.ByteBuffer; +import java.nio.channels.SocketChannel; +import java.util.HashMap; import java.util.concurrent.RejectedExecutionException; import java.util.logging.Logger; +import org.mmocore.network.IAcceptFilter; import org.mmocore.network.IClientFactory; import org.mmocore.network.IMMOExecutor; import org.mmocore.network.IPacketHandler; @@ -29,6 +33,8 @@ import com.l2jserver.gameserver.ThreadPoolManager; import com.l2jserver.gameserver.network.L2GameClient.GameClientState; import com.l2jserver.gameserver.network.clientpackets.*; +import com.l2jserver.loginserver.SelectorHelper; +import com.l2jserver.loginserver.SelectorHelper.Flood; import com.l2jserver.util.Util; /** @@ -40,9 +46,10 @@ * Note: If for a given exception a packet needs to be handled on more then one state, then it should be added to all these states. * @author KenM */ -public final class L2GamePacketHandler implements IPacketHandler<L2GameClient>, IClientFactory<L2GameClient>, IMMOExecutor<L2GameClient> +public final class L2GamePacketHandler implements IPacketHandler<L2GameClient>, IClientFactory<L2GameClient>, IMMOExecutor<L2GameClient>, IAcceptFilter { private static final Logger _log = Logger.getLogger(L2GamePacketHandler.class.getName()); + private final HashMap<Integer, Flood> _ipFloodMap = new HashMap<Integer, Flood>(); // implementation public ReceivablePacket<L2GameClient> handlePacket(ByteBuffer buf, L2GameClient client) @@ -1130,4 +1137,52 @@ } } } + + @Override + public boolean accept(SocketChannel sc) + { + InetAddress addr = sc.socket().getInetAddress(); + int h = SelectorHelper.hash(addr.getAddress()); + + long current = System.currentTimeMillis(); + Flood f; + synchronized (_ipFloodMap) + { + f = _ipFloodMap.get(h); + } + if (f != null) + { + if (f.trys == -1) + { + f.lastAccess = current; + return false; + } + + if (f.lastAccess + 1000 > current) + { + f.lastAccess = current; + + if (f.trys >= 3) + { + f.trys = -1; + return false; + } + + f.trys++; + } + else + { + f.lastAccess = current; + } + } + else + { + synchronized (_ipFloodMap) + { + _ipFloodMap.put(h, new Flood()); + } + } + + return true; + } } Index: java/com/l2jserver/loginserver/SelectorHelper.java =================================================================== --- java/com/l2jserver/loginserver/SelectorHelper.java (revision 3728) +++ java/com/l2jserver/loginserver/SelectorHelper.java (working copy) @@ -123,18 +123,18 @@ * @param ip * @return */ - private int hash(byte[] ip) + public static int hash(byte[] ip) { return ip[0] & 0xFF | ip[1] << 8 & 0xFF00 | ip[2] << 16 & 0xFF0000 | ip[3] << 24 & 0xFF000000; } - private class Flood + public static class Flood { - long lastAccess; - int trys; + public long lastAccess; + public int trys; - Flood() + public Flood() { lastAccess = System.currentTimeMillis(); trys = 0;
-
[UPDATED-Share]New event - Biohazard
streamss replied to azizilaika's topic in Server Shares & Files [L2J]
see my post plz, i'm included all and transform.too. -
[UPDATED-Share]New event - Biohazard
streamss replied to azizilaika's topic in Server Shares & Files [L2J]
fix: Index: net/sf/l2j/gameserver/model/actor/instance/L2PcInstance.java =================================================================== --- net/sf/l2j/gameserver/model/actor/instance/L2PcInstance.java (revision 3695) +++ net/sf/l2j/gameserver/model/actor/instance/L2PcInstance.java (working copy) @@ -137,6 +137,7 @@ import net.sf.l2j.gameserver.model.entity.L2Event; import net.sf.l2j.gameserver.model.entity.Siege; import net.sf.l2j.gameserver.model.entity.TvTEvent; +import net.sf.l2j.gameserver.model.entity.Biohazard; import net.sf.l2j.gameserver.model.itemcontainer.Inventory; import net.sf.l2j.gameserver.model.itemcontainer.ItemContainer; import net.sf.l2j.gameserver.model.itemcontainer.PcFreight; @@ -309,6 +310,7 @@ }; private static final int[] COMMON_CRAFT_LEVELS = { 5, 20, 28, 36, 43, 49, 55, 62 }; + private static boolean _inBiohazard = false; public class AIAccessor extends L2Character.AIAccessor { @@ -510,6 +512,16 @@ private int _obsZ; private boolean _observerMode = false; + public boolean _isZombie = false; + public void setIsZombie(boolean a) + { + _isZombie = a; + } + public boolean isZombie() + { + return _isZombie; + } + /** Stored from last ValidatePosition **/ private Point3D _lastServerPosition = new Point3D(0, 0, 0); @@ -4893,6 +4905,8 @@ public void untransform() { + if (inBiohazard() && Biohazard.isStarted() && _transformation != null && isZombie()) + return; if (_transformation != null) { setTransformAllowedSkills(new int[]{}); @@ -5333,6 +5347,22 @@ // Kill the L2PcInstance if (!super.doDie(killer)) return false; + + if (killer instanceof L2PcInstance) + { + L2PcInstance pl = (L2PcInstance) killer; + if (inBiohazard() && !isZombie() && pl.inBiohazard() && pl.isZombie() && Biohazard.isStarted()) + { + pl.abortAttack(); + pl.abortCast(); + doRevive(); + Biohazard.infectPlayer(this); + stopAllEffects(); + setCurrentHp(getMaxHp()); + setCurrentMp(getMaxMp()); + setCurrentCp(getMaxCp()); + } + } if (isMounted()) stopFeed(); @@ -5450,6 +5480,17 @@ { reviveRequest(this, null, false); } + + if (isZombie() && inBiohazard()) + { + if(Biohazard._infected.contains(this)) + { + Biohazard._infected.remove(this); + untransform(); + if (Biohazard._infected.size() == 0) + Biohazard.playersWin(); + } + } return true; } @@ -8399,7 +8440,16 @@ // Check if the attacker isn't the L2PcInstance Pet if (attacker == this || attacker == getPet()) return false; - + + L2PcInstance player = null; + if (attacker instanceof L2PcInstance) + player = (L2PcInstance) attacker; + if (attacker instanceof L2SummonInstance) + player = ((L2SummonInstance) attacker).getOwner(); + + if (player != null) + if (Biohazard.isStarted() && player.inBiohazard() && inBiohazard() && player.isZombie() != isZombie()) + return true; // TODO: check for friendly mobs // Check if the attacker is a L2MonsterInstance if (attacker instanceof L2MonsterInstance) @@ -8788,16 +8838,35 @@ return false; } + if (skill.getId() == 619 && inBiohazard() && isZombie()) + return false; + //************************************* Check Skill Type ******************************************* - // Check if this is offensive magic skill if (skill.isOffensive()) { - if ((isInsidePeaceZone(this, target)) && !getAccessLevel().allowPeaceAttack()) + if ((isInsidePeaceZone(this, target)) && !getAccessLevel().allowPeaceAttack()) { // If L2Character or target is in a peace zone, send a system message TARGET_IN_PEACEZONE a Server->Client packet ActionFailed sendPacket(new SystemMessage(SystemMessageId.TARGET_IN_PEACEZONE)); sendPacket(ActionFailed.STATIC_PACKET); + + boolean cond = true; + L2PcInstance trgtF = null; + if (target instanceof L2PcInstance) + trgtF = (L2PcInstance)target; + else if (target instanceof L2SummonInstance) + trgtF = ((L2SummonInstance)target).getOwner(); + if (trgtF != null) + if (Biohazard.isStarted() && trgtF.inBiohazard() && inBiohazard()) + { + if (trgtF.isZombie() != isZombie()) + cond = true; + if (trgtF.isZombie() == isZombie()) + cond = false; + } + + if (!cond) return false; } @@ -9213,6 +9282,8 @@ { if(skill.isPvpSkill()) // pvp skill { + if (Biohazard.isStarted() && inBiohazard() && ((L2PcInstance)target).inBiohazard() && isZombie() != ((L2PcInstance)target).isZombie()) + return true; if(getClan() != null && ((L2PcInstance)target).getClan() != null) { if(getClan().isAtWarWith(((L2PcInstance)target).getClan().getClanId()) && ((L2PcInstance)target).getClan().isAtWarWith(getClan().getClanId())) @@ -9243,6 +9314,10 @@ return true; } + private boolean inBiohazard() { + + return _inBiohazard; + } /** * Return True if the L2PcInstance is a Mage.<BR><BR> */ @@ -11542,7 +11617,16 @@ { _log.log(Level.SEVERE, "deleteMe()", e); } - + + try + { + Biohazard.onLogout(this); + } + catch (Exception e) + { + _log.log(Level.SEVERE, "deleteMe()", e); + } + // Update database with items in its inventory and remove them from the world try { @@ -12774,6 +12858,9 @@ private boolean _canFeed; private int _afroId = 0; private boolean _isInSiege; + public int _oldX; + public int _oldY; + public int _oldZ; public Collection<TimeStamp> getReuseTimeStamps() { @@ -13976,4 +14063,8 @@ break; } } + public void setIsInBiohazard(boolean b) { + + _inBiohazard = b; + } } \ No newline at end of file Index: net/sf/l2j/gameserver/model/entity/Biohazard.java =================================================================== --- net/sf/l2j/gameserver/model/entity/Biohazard.java (revision 0) +++ net/sf/l2j/gameserver/model/entity/Biohazard.java (revision 0) @@ -0,0 +1,288 @@ +/* + * This program is free software: you can redistribute it and/or modify it under + * the terms of the GNU General Public License as published by the Free Software + * Foundation, either version 3 of the License, or (at your option) any later + * version. + * + * This program is distributed in the hope that it will be useful, but WITHOUT + * ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS + * FOR A PARTICULAR PURPOSE. See the GNU General Public License for more + * details. + * + * You should have received a copy of the GNU General Public License along with + * this program. If not, see <http://www.gnu.org/licenses/>. + */ +package net.sf.l2j.gameserver.model.entity; + +import java.util.Calendar; +import java.util.logging.Logger; + +import javolution.util.FastSet; +import net.sf.l2j.gameserver.Announcements; +import net.sf.l2j.gameserver.instancemanager.TransformationManager; +import net.sf.l2j.gameserver.model.L2World; +import net.sf.l2j.gameserver.model.actor.instance.L2PcInstance; +import net.sf.l2j.gameserver.model.olympiad.Olympiad; +import net.sf.l2j.gameserver.network.serverpackets.PlaySound; +import net.sf.l2j.gameserver.util.Broadcast; +import net.sf.l2j.util.Rnd; + +/** + * @author Laikeriz + */ +public class Biohazard +{ + enum EventState + { + INACTIVE, + INACTIVATING, + REGISTERING, + STARTED, + REWARDING + } + + private final static Logger _log = Logger.getLogger(Biohazard.class.getName()); + private static EventState _state = EventState.INACTIVE; + + public static FastSet<L2PcInstance> _participants = new FastSet<L2PcInstance>(); + public static FastSet<L2PcInstance> _infected = new FastSet<L2PcInstance>(); + public static FastSet<L2PcInstance> _notInfected = new FastSet<L2PcInstance>(); + + public static boolean isStarted() + { + if (_state == EventState.STARTED) + return true; + return false; + } + + public static boolean isRegistering() + { + if (_state == EventState.REGISTERING) + return true; + return false; + } + + public static void startRegistering() + { + if (_state == EventState.REGISTERING || _state == EventState.STARTED) + return; + Announcements.getInstance().announceToAll("Biohazard: Registration is open."); + Announcements.getInstance().announceToAll("Biohazard: Type \".bhreg\" to register to event."); + Announcements.getInstance().announceToAll("Biohazard: Type \".bhunreg\" to unregister from event."); + _state = EventState.REGISTERING; + int step = 0; + int after = 0; + for (int i = 40; i > 0; i-=10) + { + switch(i) + { + case 40: + step = 5; + after = 15; + break; + case 30: + step = 5; + after = 10; + break; + case 20: + step = 4; + after = 5; + break; + case 10: + step = 1; + after = 1; + break; + } + if (_state == EventState.INACTIVE) + return; + Announcements.getInstance().announceToAll("Biohazard: Registration will be closed in " + after + " minute(s)."); + try{Thread.sleep(step*60000);}catch (Exception e){} + } + //sound = ""; + try{Thread.sleep(60000);}catch (Exception e){} + if (_state == EventState.INACTIVE) + return; + if (_participants.size() >= 2) + { + Announcements.getInstance().announceToAll("Biohazard: Teleporting players in 20 seconds."); + try{Thread.sleep(20000);}catch (Exception e){} + _state = EventState.STARTED; + startEventAndTelePl(); + } + else + Announcements.getInstance().announceToAll("Biohazard: Event aborted due to lack of participants."); + } + + public static void addParticipant(L2PcInstance player) + { + if (Olympiad.getInstance().isRegistered(player) || TvTEvent.isPlayerParticipant(player.getObjectId())) + { + player.sendMessage("You cannot register because of registration in another event"); + return; + } + if (_state == EventState.REGISTERING && !_participants.contains(player)) + { + _participants.add(player); + player.sendMessage("You have successfully registered to this event"); + } + else + player.sendMessage("You are already registered or it's not registration time."); + } + + public static void removeParticipant(L2PcInstance player) + { + if (_state == EventState.REGISTERING) + { + if (_participants.contains(player)) + _participants.remove(player); + else + player.sendMessage("You aren't registered in this event."); + player.setIsInBiohazard(false); + } + else + player.sendMessage("It's not registration time."); + } + public static void startEventAndTelePl() + { + if (_state == EventState.INACTIVE) + return; + synchronized(_participants) + { + for (L2PcInstance pl : _participants) + if (pl.isOnline() == 1) + { + _notInfected.add(pl); + pl._oldX = pl.getX(); + pl._oldY = pl.getY(); + pl._oldZ = pl.getZ(); + pl.teleToLocation(-17507,143206,-3911); + pl.setTeam(0); + pl.setIsInBiohazard(true); + pl.untransform(); + } + } + Announcements.getInstance().announceToAll("Biohazard: Teleportion done."); + Announcements.getInstance().announceToAll("Biohazard: One player was infected by untreatable virus!"); + Announcements.getInstance().announceToAll("Biohazard: In about one minute virus will take over his body and he will become zombie!"); + try{Thread.sleep(60000);}catch (Exception e){} + int num = Math.round(Rnd.get(_notInfected.size()-1)); + L2PcInstance infectFirst = ((L2PcInstance[])getAllNotInfected())[num]; + infectPlayer(infectFirst); + Announcements.getInstance().announceToAll("Biohazard: Virus took over " + infectFirst.getName() + " body and he wants to infect everybody else!"); + } + + public static void infectPlayer(L2PcInstance zombie) + { + if (_state == EventState.INACTIVE) + return; + if (zombie.isTransformed()) + zombie.untransform(); + zombie.setIsZombie(true); + _notInfected.remove(zombie); + _infected.add(zombie); + TransformationManager.getInstance().transformPlayer(303, zombie); + if (_notInfected.size() == 0) + zombiesWin(); + } + + public static void onLogout(L2PcInstance playerInstance) + { + if (_state == EventState.REGISTERING) + removeParticipant(playerInstance); + else if (_state == EventState.STARTED) + { + playerInstance.setXYZ(playerInstance._oldX,playerInstance._oldY,playerInstance._oldZ); + if (!playerInstance.isZombie()) + _notInfected.remove(playerInstance); + else if (playerInstance.isZombie()) + _infected.remove(playerInstance); + if (_notInfected.size() == 0) + zombiesWin(); + if (_infected.size() == 0) + playersWin(); + } + } + + public static void zombiesWin() + { + if (_state == EventState.INACTIVE) + return; + Announcements.getInstance().announceToAll("Biohazard: Zombies won."); + Announcements.getInstance().announceToAll("Biohazard: Rewarding and teleporting participants back to village in 20 seconds."); + _state = EventState.REWARDING; + try{Thread.sleep(20000);}catch (Exception e){} + synchronized(_infected) + { + for (L2PcInstance pl : _infected) + if (pl.isOnline() == 1) + pl.addItem("Biohazard", 6673, 1, pl, true); + } + synchronized(_participants) + { + for (L2PcInstance pl : _participants) + if (pl.isOnline() == 1) + { + pl.teleToLocation(pl._oldX,pl._oldY,pl._oldZ); + pl.setIsInBiohazard(false); +// if (pl.inWorld() == 1) +// pl.setTeam(pl.getFactionId()); + if (pl.isTransformed()) + pl.untransform(); + } + } + _participants.clear(); + _infected.clear(); + _notInfected.clear(); + _state = EventState.INACTIVE; + } + + public static void playersWin() + { + Announcements.getInstance().announceToAll("Biohazard: Players won."); + Announcements.getInstance().announceToAll("Biohazard: Rewarding and teleporting participants back to village in 20 seconds."); + _state = EventState.REWARDING; + try{Thread.sleep(20000);}catch (Exception e){} + synchronized(_notInfected) + { + for (L2PcInstance pl : _notInfected) + if (pl.isOnline() == 1) + { + pl.addItem("Biohazard", 6673, 1, pl, true); + } + } + synchronized(_participants) + { + for (L2PcInstance pl : _participants) + if (pl.isOnline() == 1) + { + pl.teleToLocation(pl._oldX,pl._oldY,pl._oldZ); + pl.setIsInBiohazard(false); +// if (pl.inWorld() == 1) +// pl.setTeam(pl.getFactionId()); + if (pl.isTransformed()) + pl.untransform(); + } + } + _participants.clear(); + _infected.clear(); + _notInfected.clear(); + _state = EventState.INACTIVE; + } + + public static L2PcInstance[] getAllNotInfected() + { + synchronized(_notInfected) + { + return _notInfected.toArray(new L2PcInstance[_notInfected.size()]); + } + } + + public static void abortEvent() + { + _state = EventState.INACTIVE; + _participants.clear(); + _notInfected.clear(); + _infected.clear(); + Announcements.getInstance().announceToAll("Biohazard: Event aborted."); + } +} \ No newline at end of file #P voicedcommandhandlers Index: castle.java =================================================================== --- castle.java (revision 6775) +++ castle.java (working copy) @@ -19,6 +19,7 @@ import net.sf.l2j.gameserver.model.actor.instance.L2DoorInstance; import net.sf.l2j.gameserver.model.actor.instance.L2PcInstance; import net.sf.l2j.gameserver.model.entity.Castle; +import net.sf.l2j.gameserver.model.entity.Biohazard; /** * @@ -30,7 +31,9 @@ { "open doors", "close doors", - "ride wyvern" + "ride wyvern", + "bhreg", + "bhunreg" }; /** @@ -70,6 +73,10 @@ activeChar.mount(12621, 0, true); } } + else if (command.equalsIgnoreCase("bhreg")) + Biohazard.addParticipant(playerInstance); + else if (command.equalsIgnoreCase("bhunreg")) + Biohazard.removeParticipant(playerInstance); return true; } Index: AdminEventEngine.java =================================================================== --- AdminEventEngine.java (revision 6775) +++ AdminEventEngine.java (working copy) @@ -34,6 +34,7 @@ import net.sf.l2j.gameserver.model.actor.L2Npc; import net.sf.l2j.gameserver.model.actor.instance.L2PcInstance; import net.sf.l2j.gameserver.model.entity.L2Event; +import net.sf.l2j.gameserver.model.entity.Biohazard; import net.sf.l2j.gameserver.network.serverpackets.CharInfo; import net.sf.l2j.gameserver.network.serverpackets.ExBrExtraUserInfo; import net.sf.l2j.gameserver.network.serverpackets.ItemList; @@ -74,7 +75,9 @@ "admin_event_control_unpoly", "admin_event_control_prize", "admin_event_control_chatban", - "admin_event_control_finish" + "admin_event_control_finish", + "admin_bh_start", + "admin_bh_abort" }; private static String tempBuffer = ""; @@ -90,6 +93,14 @@ { showNewEventPage(activeChar); } + else if (command.equals("admin_bh_start")) + { + Biohazard.startRegistering(); + } + else if (command.equals("admin_bh_abort")) + { + Biohazard.abortEvent(); + } else if (command.startsWith("admin_add")) { tempBuffer += command.substring(10); And SQL part : [code=text]INSERT INTO `admin_command_access_rights` VALUES ('admin_bh_abort', '1'); INSERT INTO `admin_command_access_rights` VALUES ('admin_bh_start', '1'); [/code] -
LordPE -> PE Editor -> путь к l2.exe -> Directories -> Import Table "..." -> add Import... ->Dll: DllApp API: mafey -> Ok http://dump.ru/file/3574524 pass:la2base.ru who can make this better?
-
Thx, you fix for exploits - very cool [javac] REPLACE\L2_GameServer\java\net\sf\l2j\gameserver\network\cl ientpackets\MoveBackwardToLocation.java:85: cannot find symbol [javac] symbol : variable player [javac] location: class net.sf.l2j.gameserver.network.clientpackets.MoveBack wardToLocation [javac] if (!FloodProtector.getInstance().tryPerformAction(player.getO bjectId(), FloodProtector.PROTECTED_PACKETS)) [javac] ^ [javac] REPLACE\L2_GameServer\java\net\sf\l2j\gameserver\network\cl ientpackets\MoveBackwardToLocation.java:85: cannot find symbol [javac] symbol : variable FloodProtector [javac] location: class net.sf.l2j.gameserver.network.clientpackets.MoveBack wardToLocation [javac] if (!FloodProtector.getInstance().tryPerformAction(player.getO bjectId(), FloodProtector.PROTECTED_PACKETS)) [javac] ^ [javac] REPLACE\L2_GameServer\java\net\sf\l2j\gameserver\network\cl ientpackets\MoveBackwardToLocation.java:85: cannot find symbol [javac] symbol : variable FloodProtector [javac] location: class net.sf.l2j.gameserver.network.clientpackets.MoveBack wardToLocation [javac] if (!FloodProtector.getInstance().tryPerformAction(player.getO bjectId(), FloodProtector.PROTECTED_PACKETS)) [javac] ^ [javac] 3 errors
-
maybe screens and upload on 4share?
-
[Share] Lineage2 Websites + (PDS+php scripts) Included !
streamss replied to GoDofAdeN's topic in Off-Topics
GoDofAdeN wow..nice..it's very beautiful -
Once your apply the core part, no one can't use most of the reinforcement skills, and toggle skills by the normal methods. It doesn't matter if you are in the event, or in a town, or in a arena, the only way to use those skills is pressing SHIFT + click. how i can fix this
-
[Share] Retail Fence Event (Walls)
streamss replied to DjStereo's topic in Server Shares & Files [L2J]
retail from l2jserver forum -
[Share]Clan community board of off servers for java
streamss replied to Emrys's topic in Server Shares & Files [L2J]
nice, but..on off in community i have auction -
[Share] New Template For PHP Fusion By Me!
streamss replied to iPwned's topic in Server Development Discussion [L2J]
design bad... -
thx. nice share
-
[Share] Advanced Hitman (Global Daily Event)
streamss replied to Setekh's topic in Server Shares & Files [L2J]
You can not view this topic because you do not have eneough posts man... hm, i see only screens and nothing more -
[Guide] How to increase lineage2 performance
streamss replied to 1tm's topic in Client Development Discussion
really help -
in this patch work all correctly?
-
[UPDATED-Share]New event - Biohazard
streamss replied to azizilaika's topic in Server Shares & Files [L2J]
author, can you help me? plz -
[Share] Advanced Hitman (Global Daily Event)
streamss replied to Setekh's topic in Server Shares & Files [L2J]
can you upload screens? -
[Share] Server Bypass Protection.
streamss replied to SySt3MGaM3RFr3aKs's topic in Server Shares & Files [L2J]
# ServerBypass - server bypass flooding FloodProtectorServerBypassInterval = 5 FloodProtectorServerBypassLogFlooding = False FloodProtectorServerBypassPunishmentLimit = 0 FloodProtectorServerBypassPunishmentType = none FloodProtectorServerBypassPunishmentTime = 0