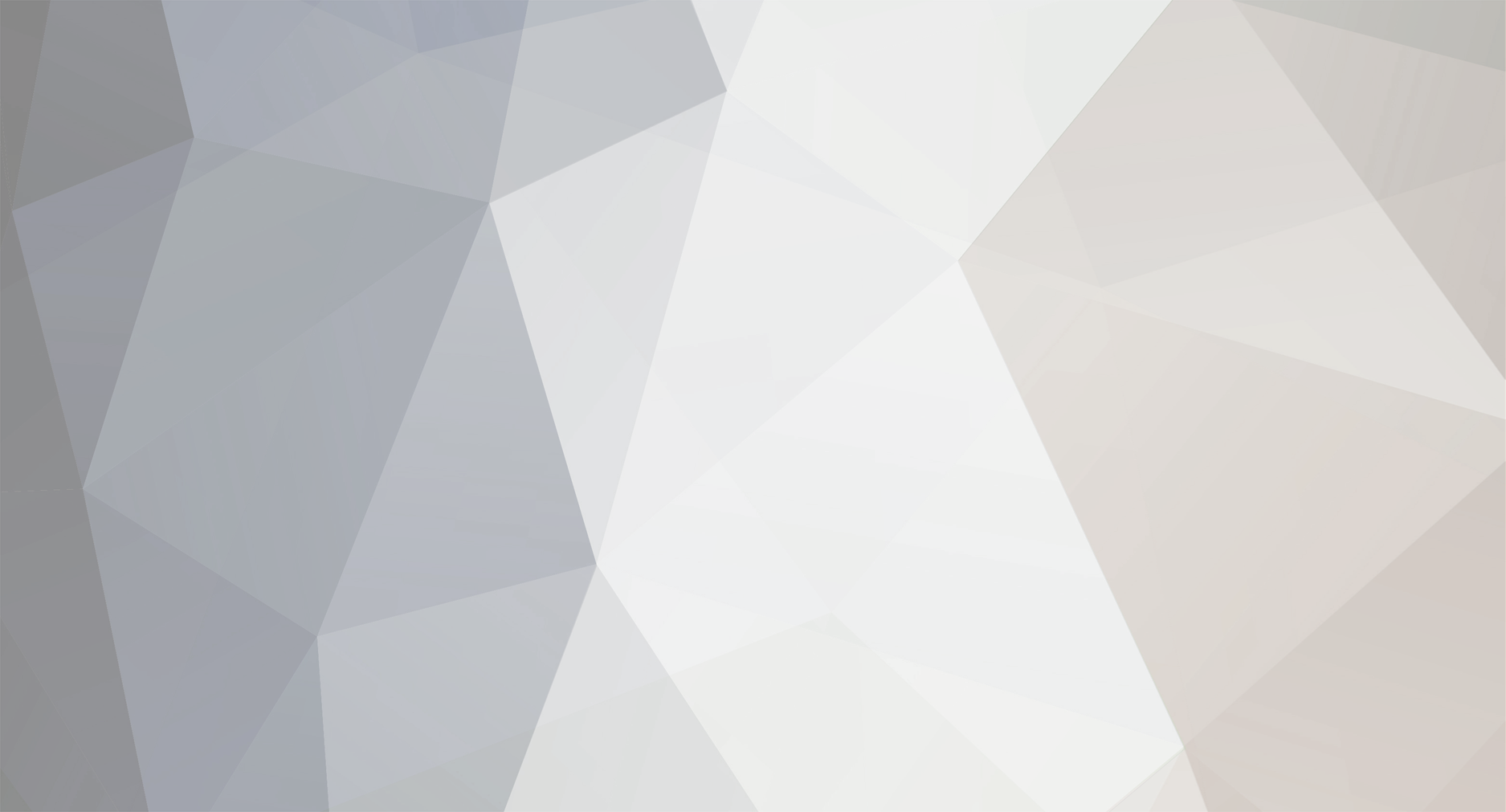
alextoti99
-
Posts
105 -
Credits
0 -
Joined
-
Last visited
-
Feedback
0%
Content Type
Articles
Profiles
Forums
Store
Posts posted by alextoti99
-
-
ennousa na ftiaxoume project opws px o l2jfrozen oxi na kanoume server -.-
-
den katalaba ti e3oda
-
3erw gi auto lew k alla atoma ama boroun na me boi8isoun gt dn ta gnwrizw olla fisikaY
Y.y an kanoume to project na einai free (oti 8a pei to team)
-
3erw arketa java html k xml gia ta stats
-
enna apo ta duo dn eiparxei 8ema :D ama 8elete peite m i gracia final i high five
-
Na ginei ena kalo project gia gracia final gt dn eiparxei olloi kanoun interlude alla polloi endiaferonte k gia alla clients
-
Geia sas skeftome me kkati filous m na ftiaxoume ena gracia final project. Opoios 8elei na simetexei as grapei apo katw
-
Hello i tried to make it for interlude frozen code:
package custom.PartyTeleporter; import com.l2jfrozen.gameserver.cache.HtmCache; import com.l2jfrozen.gameserver.datatables.sql.ItemTable; import com.l2jfrozen.gameserver.model.L2Character; import com.l2jfrozen.gameserver.model.L2Party; import com.l2jfrozen.gameserver.model.L2TeleportLocation; import com.l2jfrozen.gameserver.model.actor.instance.L2PcInstance; import com.l2jfrozen.gameserver.model.quest.Quest; import com.l2jfrozen.gameserver.model.zone.L2ZoneType; import com.l2jfrozen.gameserver.network.serverpackets.InventoryUpdate; import com.l2jfrozen.gameserver.network.serverpackets.ItemList; import com.l2jfrozen.gameserver.network.serverpackets.NpcHtmlMessage; import com.l2jfrozen.gameserver.network.serverpackets.StatusUpdate; /** * @author `Heroin * Made For Maxcheaters.com * PartyTeleporter */ public class PartyTeleporter extends Quest { private static final int npcid = 36650; // npc id //------------------------------------- //Teleport Location Coordinates X,Y,Z. //Use /loc command in game to find them. private static final int locationX = -56742; // npc id private static final int locationY = 140569; // npc id private static final int locationZ = -2625; // npc id //------------------------------------- //------------------------------------- // Select the id of your zone. // If you dont know how to find your zone id is simple. // Go to data/zones/(your zone file).xml and find your zone // E.g: <zone name="dion_monster_pvp" id="6" type="ArenaZone" shape="NPoly" minZ="-3596" maxZ="0"> /**The id of your zone is id="6" */ /**---------------------------------------------------------------------------*/ /**WARNING: If your zone does not have any id or your location is not on any zone in data/zones/ folder, you have to add one by your self*/ // required to calculate parties & players /**---------------------------------------------------------------------------*/ private static final int ZoneId = 155; //Here you have to set your zone Id //------------------------------------- private static final int MinPtMembers = 2; // Minimum Party Members Count For Enter on Zone. private static final int ItemConsumeId = 57; // Item Consume id. private static final int ItemConsumeNum = 100; // Item Consume Am.ount. private static final boolean ShowPlayersInside = true; //If you set it true, NPC will show how many players are inside area. private static final boolean ShowPartiesInside = true; //If you set it true, NPC will show how many parties are inside area. //------------------------------------- private static String htm = "data/scripts/custom/PartyTeleporter/1.htm"; //html location. private static String ItemName = ItemTable.getInstance().createDummyItem(ItemConsumeId).getItemName(); //Item name, Dont Change this public PartyTeleporter(int questId, String name, String descr) { super(questId, name, descr); addFirstTalkId(npcid); addTalkId(npcid); addStartNpc(npcid); } @Override public String onAdvEvent(String event, L2NpcInstance npc, L2PcInstance player) { if (event.startsWith("partytp")) { TP(event, npc, player, event); } return ""; } @SuppressWarnings("deprecation") public int getPartiesInside(int zoneId)//Calculating parties inside party area. { int i = 0; for (L2ZoneType zone : L2TeleportLocation.getInstance().getAllZones()) if (zone.getId() == zoneId) { for (L2Character character : zone.getCharactersInside().values()) if (character instanceof L2PcInstance && (!((L2PcInstance) character).getClient().isDetached()) && ((L2PcInstance) character).getParty() != null && ((L2PcInstance) character).getParty().isLeader((L2PcInstance) character)) i++; } return i; } @SuppressWarnings("deprecation") public int getPlayerInside(int zoneId)//Calculating players inside party area. { int i = 0; for (L2ZoneType zone : ZoneManager.getInstance().getAllZones()) if (zone.getId() == zoneId) { for (L2Character character : zone.getCharactersInside().values()) if (character instanceof L2PcInstance && (!((L2PcInstance) character).getClient().isDetached())) i++; } return i; } private boolean PartyItemsOk(L2PcInstance player) //Checks if all party members have the item in their inventory. //If pt member has not enough items, party not allowed to enter. { try { for (L2PcInstance member : player.getParty().getPartyMembers()) { if (member.getInventory().getItemByItemId(ItemConsumeId) == null) { player.sendMessage("Your party member "+member.getName()+" does not have enough items."); return false; } if (member.getInventory().getItemByItemId(ItemConsumeId).getCount() < ItemConsumeNum) { player.sendMessage("Your party member "+member.getName()+" does not have enough items."); return false; } } return true; } catch (Exception e) { player.sendMessage("Something went wrong try again."); return true; } } private void proccessTP(L2PcInstance player) // Teleporting party members to zone { for (L2PcInstance member : player.getParty().getPartyMembers()) { member.teleToLocation(locationX, locationY, locationZ);//Location X, Y ,Z } } private void TP(String event, L2NpcInstance npc, L2PcInstance player, String command) // Teleport player & his party { try { L2Party pt = player.getParty(); if (pt == null) { player.sendMessage("You are not currently on party."); return; } if (!pt.isLeader(player)) { player.sendMessage("You are not party leader."); return; } if (pt.getMemberCount() < MinPtMembers) { player.sendMessage("You are going to need a bigger party " + "in order to enter party area."); return; } if (!PartyItemsOk(player)) { return; } else { proccessTP(player); for (L2PcInstance ppl : pt.getPartyMembers()) { if (ppl.getObjectId() != player.getObjectId())//Dont send this message to pt leader. { ppl.sendMessage("Your party leader asked to teleport on party area!");//Message only to party members } ppl.sendMessage(ItemConsumeNum+" "+ItemName+" have been dissapeared.");//Item delete from inventory message ppl.getInventory().destroyItemByItemId("Party_Teleporter", ItemConsumeId, ItemConsumeNum, ppl, true);//remove item from inventory ppl.sendPacket(new InventoryUpdate());//Update ppl.sendPacket(new ItemList(ppl, false));//Update ppl.sendPacket(new StatusUpdate(ppl));//Update } //Sends message to party leader. player.sendMessage(ItemConsumeNum*player.getParty().getMemberCount()+" "+ItemName+" dissapeard from your party."); } } catch (Exception e) { player.sendMessage("Something went wrong try again."); } } @Override public String onFirstTalk(L2NpcInstance npc, L2PcInstance player) { final int npcId = npc.getNpcId(); if (player.getQuestState(getName()) == null) { newQuestState(player); } if (npcId == npcid) { String html = HtmCache.getInstance().getHtm(player.getHtmlPrefix(), htm); html = html.replaceAll("%player%", player.getName());//Replaces %player% with player name on html html = html.replaceAll("%itemname%", ItemName);//Item name replace on html html = html.replaceAll("%price%", player.getParty()!=null ? ""+ItemConsumeNum*player.getParty().getMemberCount()+"": "0");//Price calculate replace html = html.replaceAll("%minmembers%", ""+MinPtMembers);//Mimum entry party members replace html = html.replaceAll("%allowed%", isAllowedEnter(player) ? "<font color=00FF00>allowed</font>" : "<font color=FF0000>not allowed</font>");//Condition checker replace on html html = html.replaceAll("%parties%", ShowPartiesInside ? "<font color=FFA500>Parties Inside: "+getPartiesInside(ZoneId)+"</font><br>": "");//Parties inside html = html.replaceAll("%players%", ShowPlayersInside ? "<font color=FFA500>Players Inside: "+getPlayerInside(ZoneId)+"</font><br>": "");//Players Inside NpcHtmlMessage npcHtml = new NpcHtmlMessage(0); npcHtml.setHtml(html); player.sendPacket(npcHtml); } return ""; } private boolean isAllowedEnter(L2PcInstance player) //Checks if player & his party is allowed to teleport. { if (player.getParty() != null) { if( player.getParty().getMemberCount() >= MinPtMembers && PartyItemsOk(player))//Party Length & Item Checker { return true; } else { return false; } } else { return false; } } public static void main(final String[] args) { new PartyTeleporter(-1, PartyTeleporter.class.getSimpleName(), "custom"); System.out.println("Party Teleporter by `Heroin has been loaded successfully!"); } }
but there are still some errors
-
Server is ONLINE!
-
Why not :D
-
Hello all we are opening a new server L2 Solaris
Info:
Type: Interlude
Safe/Max: 6/25 +20 to +25 only with crystal scrolls
Normal Scroll: 60%
Blessed Scroll: 80% (when fails it goes to +6)
Crystall Scroll: 100% (only from vote shop and some raidbosses)
Xp/Sp: x5000 Donator's: x8000
Adena: x3000 Donator's: x6000
Drop/Spoil: x1 Donator's: x2
TvT,Ctf,Dm
Custom Npcs:
Gm Shop
Custom Shop (inside are Vote and PvP Shop)
Global Gatekeeper
Scheme Buffer
Boss Manager
Bug Report Manager
PassWord Changer
SubClass And Clan/Ally Manager
Custom Farm Zones
Custom RaidBosses
Solo Zone:
1 without Pk And 1 with PvPs
Party Farm Zone with PvPs and Pks
PvP/Pk Zone
Custom Recommendation Item
Custom Clan Reputation Item
Vote System (for hopzone and topzone but at our site are more vote site, without reward, if you want more pvps)
Custom Gm Help Events:
Team Vs Team
Hero Event
HnS (Hide And Seek Event)
And Many More Events Soon!
Tattoos Give Special Stats For Improved GamePlay
A big deal of bugs are fixed (we try everyday to fix everything)
Skills Are Working Perfectly
Also Our Server Is Based On Java Platform
We have host at ovh
Commands:
.castle (for sieges' registration)
.online
.repair
Many More In Game!!!
Server will open at 23/8 12 gmt+2 pm
Site: l2solaris.tk
No Custom
Also if you are a clan leader and you bring:
10 ppl 3k ancient adena and 5kkk adena
20 ppl 5k ancient adena and 1k clan reputation
30 ppl 10k clan reputation and 1 clan level
40 ppl 1 clan hall
10 ppl after 40 ppl will give 10k clan reputation more!
-
Hello all we are opening a new server L2 Solaris
Info:
Type: Interlude
Safe/Max: 6/25 +20 to +25 only with crystal scrolls
Normal Scroll: 60%
Blessed Scroll: 80% (when fails it goes to +6)
Crystall Scroll: 100% (only from vote shop and some raidbosses)
Xp/Sp: x5000 Donator's: x8000
Adena: x3000 Donator's: x6000
Drop/Spoil: x1 Donator's: x2
TvT,Ctf,Dm
Custom Npcs:
Gm Shop
Custom Shop (inside are Vote and PvP Shop)
Global Gatekeeper
Scheme Buffer
Boss Manager
Bug Report Manager
PassWord Changer
SubClass And Clan/Ally Manager
Custom Farm Zones
Custom RaidBosses
Solo Zone:
1 without Pk And 1 with PvPs
Party Farm Zone with PvPs and Pks
PvP/Pk Zone
Custom Recommendation Item
Custom Clan Reputation Item
Vote System (for hopzone and topzone but at our site are more vote site, without reward, if you want more pvps)
Custom Gm Help Events:
Team Vs Team
Hero Event
HnS (Hide And Seek Event)
And Many More Events Soon!
Tattoos Give Special Stats For Improved GamePlay
A big deal of bugs are fixed (we try everyday to fix everything)
Skills Are Working Perfectly
Also Our Server Is Based On Java Platform
We have host at ovh
Commands:
.castle (for sieges' registration)
.online
.repair
Many More In Game!!!
Server will open at 23/8 12 gmt+2 pm
Site: l2solaris.tk
No Custom
Also if you are a clan leader and you bring:
10 ppl 3k ancient adena and 5kkk adena
20 ppl 5k ancient adena and 1k clan reputation
30 ppl 10k clan reputation and 1 clan level
40 ppl 1 clan hall
10 ppl after 40 ppl will give 10k clan reputation more!
-
Hello all we are opening a new server L2 Solaris
Info:
Type: Interlude
Safe/Max: 6/25 +20 to +25 only with crystal scrolls
Normal Scroll: 60%
Blessed Scroll: 80% (when fails it goes to +6)
Crystall Scroll: 100% (only from vote shop and some raidbosses)
Xp/Sp: x5000 Donator's: x8000
Adena: x3000 Donator's: x6000
Drop/Spoil: x1 Donator's: x2
TvT,Ctf,Dm
Custom Npcs:
Gm Shop
Custom Shop (inside are Vote and PvP Shop)
Global Gatekeeper
Scheme Buffer
Boss Manager
Bug Report Manager
PassWord Changer
SubClass And Clan/Ally Manager
Custom Farm Zones
Custom RaidBosses
Solo Zone:
1 without Pk And 1 with PvPs
Party Farm Zone with PvPs and Pks
PvP/Pk Zone
Custom Recommendation Item
Custom Clan Reputation Item
Vote System (for hopzone and topzone but at our site are more vote site, without reward, if you want more pvps)
Custom Gm Help Events:
Team Vs Team
Hero Event
HnS (Hide And Seek Event)
And Many More Events Soon!
Tattoos Give Special Stats For Improved GamePlay
A big deal of bugs are fixed (we try everyday to fix everything)
Skills Are Working Perfectly
Also Our Server Is Based On Java Platform
We have host at ovh
Commands:
.castle (for sieges' registration)
.online
.repair
Many More In Game!!!
Server will open at 23/8 12 gmt+2 pm
Site: l2solaris.tk
No Custom
Also if you are a clan leader and you bring:
10 ppl 3k ancient adena and 5kkk adena
20 ppl 5k ancient adena and 1k clan reputation
30 ppl 10k clan reputation and 1 clan level
40 ppl 1 clan hall
10 ppl after 40 ppl will give 10k clan reputation more!
-
Hello all we are opening a new server L2 Solaris
Info:
Type: Interlude
Safe/Max: 6/25 +20 to +25 only with crystal scrolls
Normal Scroll: 60%
Blessed Scroll: 80% (when fails it goes to +6)
Crystall Scroll: 100% (only from vote shop and some raidbosses)
Xp/Sp: x5000 Donator's: x8000
Adena: x3000 Donator's: x6000
Drop/Spoil: x1 Donator's: x2
TvT,Ctf,Dm
Custom Npcs:
Gm Shop
Custom Shop (inside are Vote and PvP Shop)
Global Gatekeeper
Scheme Buffer
Boss Manager
Bug Report Manager
PassWord Changer
SubClass And Clan/Ally Manager
Custom Farm Zones
Custom RaidBosses
Solo Zone:
1 without Pk And 1 with PvPs
Party Farm Zone with PvPs and Pks
PvP/Pk Zone
Custom Recommendation Item
Custom Clan Reputation Item
Vote System (for hopzone and topzone but at our site are more vote site, without reward, if you want more pvps)
Custom Gm Help Events:
Team Vs Team
Hero Event
HnS (Hide And Seek Event)
And Many More Events Soon!
Tattoos Give Special Stats For Improved GamePlay
A big deal of bugs are fixed (we try everyday to fix everything)
Skills Are Working Perfectly
Also Our Server Is Based On Java Platform
We have host at ovh
Commands:
.castle (for sieges' registration)
.online
.repair
Many More In Game!!!
Server will open at 23/8 12 gmt+2 pm
Site: l2solaris.tk
No Custom
Also if you are a clan leader and you bring:
10 ppl 3k ancient adena and 5kkk adena
20 ppl 5k ancient adena and 1k clan reputation
30 ppl 10k clan reputation and 1 clan level
40 ppl 1 clan hall
10 ppl after 40 ppl will give 10k clan reputation more!
-
If you have donator's status you gain more xp-sp-adena drop-drop-spoil
-
ok euxaristw pantos
-
Hello all we are opening a new server L2 Solaris
Info:
Type: Interlude
Safe/Max: 6/25 +20 to +25 only with crystal scrolls
Normal Scroll: 60%
Blessed Scroll: 80% (when fails it goes to +6)
Crystall Scroll: 100% (only from vote shop and some raidbosses)
Xp/Sp: x5000 Donator's: x8000
Adena: x3000 Donator's: x6000
Drop/Spoil: x1 Donator's: x2
TvT,Ctf,Dm
Custom Npcs:
Gm Shop
Custom Shop (inside are Vote and PvP Shop)
Global Gatekeeper
Scheme Buffer
Boss Manager
Bug Report Manager
PassWord Changer
SubClass And Clan/Ally Manager
Custom Farm Zones
Custom RaidBosses
Solo Zone:
1 without Pk And 1 with PvPs
Party Farm Zone with PvPs and Pks
PvP/Pk Zone
Custom Recommendation Item
Custom Clan Reputation Item
Vote System (for hopzone and topzone but at our site are more vote site, without reward, if you want more pvps)
Custom Gm Help Events:
Team Vs Team
Hero Event
HnS (Hide And Seek Event)
And Many More Events Soon!
Tattoos Give Special Stats For Improved GamePlay
A big deal of bugs are fixed (we try everyday to fix everything)
Skills Are Working Perfectly
Also Our Server Is Based On Java Platform
We have host at ovh
Commands:
.castle (for sieges' registration)
.online
.repair
Many More In Game!!!
Server will open at 17/8 7 gmt+2 am (date changed)
Site: l2solaris.tk
Also if you are a clan leader and you bring:
10 ppl 3k ancient adena and 5kkk adena
20 ppl 5k ancient adena and 1k clan reputation
30 ppl 10k clan reputation and 1 clan level
40 ppl 1 clan hall
10 ppl after 40 ppl will give 10k clan reputation more!
-
Geia sas xreiazome ena guide na ma8w java an ginete parakalw grapste apo katw
-
-
Hello we make a new clan TheDooms if you want to join just write down and we will tell you more!
-
Hello Here are L2 Souls Features:
Soon Online!
Site: Click Me
Info:
Xp-Sp: x5000
Drop-Spoil: x1 (for donators x2)
Adena: x4000 (for donators x5000)
Instant level 80
Subbclass level 80
Custom Armors: Abyssal
Custom Weapons: Dynasty
Custom Leveled Tattoos: LvL1 and LvL2 (for fighter and mage)
Events: Tvt Ctf Dm RaceEvent And HiddenBastard (like Hide And Seek Event)
Enchant: Safe +5
Enchant: Max +20
Enchant: Blessed Max +16
Enchant: Cystall Max +20
Conquerable Engine For Clan (like clan wars) every (To be announced)
Hero Item For Ever
Hero Item Until Restart
Noble Item For Ever
Donator Item For EverCustom Npc:
Gm Shop
Custom Shop (pvp vote and event shop inside)
Buffer Gatekeeper
Bug Report Manager
And Much More Npcs
Also Donators Take Some Skills!
Augment System Is 1+1
Have Fun! :D :D :D :D -
Stile k edw
dn katalaba
-
to stelnw afoy k einai se project
-
I need feedback to make it better
Gracia Final Project
in Server Development Discussion [Greek]
Posted
:P dn prz pantws opoioi 8elete k boreite peite m