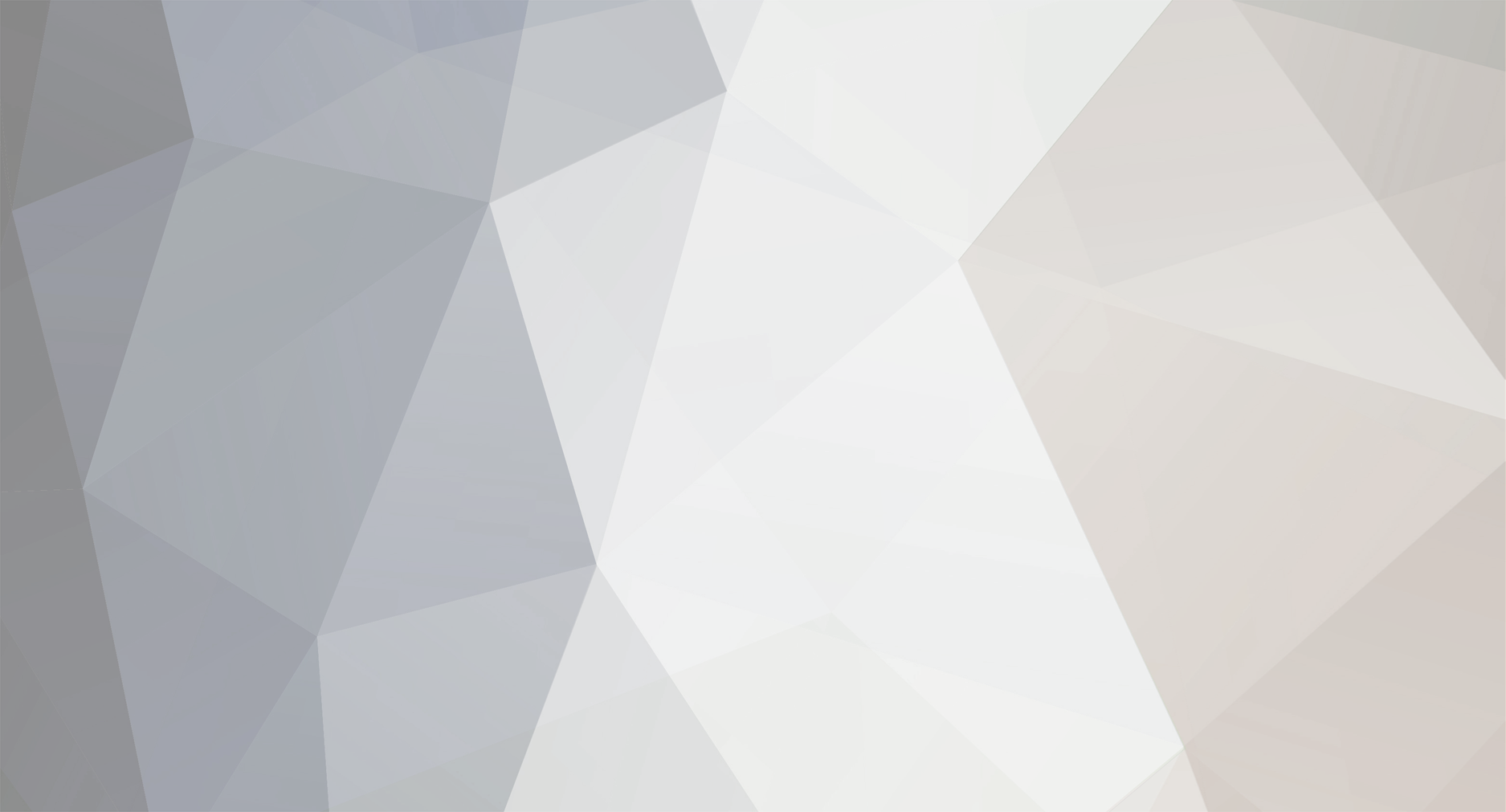
TouchAndDie
Members-
Posts
115 -
Credits
0 -
Joined
-
Last visited
-
Feedback
0%
Content Type
Articles
Profiles
Forums
Store
Everything posted by TouchAndDie
-
[fixed] hitman reward
TouchAndDie replied to TouchAndDie's question in Request Server Development Help [L2J]
i get warning :D i must use client.sendmessage :p and i have to use else if(client.getItemByItemId(9143) < bounty), if not when a player will add a contract it won't take his golden apiga... but i don't know why i get warning :| -
[fixed] hitman reward
TouchAndDie replied to TouchAndDie's question in Request Server Development Help [L2J]
i have used assassin.addItem("Hitman", 9143, pta.getBounty(), target, true); but now i don't know how to change that: else if(client.getAdena() < bounty) { client.sendMessage("Not enough Golden Apiga."); return; } -
[fixed] hitman reward
TouchAndDie replied to TouchAndDie's question in Request Server Development Help [L2J]
and what can i do with else if(client.getAdena() < bounty) { client.sendMessage("Not enough Adena."); return; } i tried to put getItemByItemId but i get warning. and this too :| client.reduceAdena("Hitman", bounty, client, true); -
[fixed] hitman reward
TouchAndDie replied to TouchAndDie's question in Request Server Development Help [L2J]
/* * This program is free software: you can redistribute it and/or modify it under * the terms of the GNU General Public License as published by the Free Software * Foundation, either version 3 of the License, or (at your option) any later * version. * * This program is distributed in the hope that it will be useful, but WITHOUT * ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS * FOR A PARTICULAR PURPOSE. See the GNU General Public License for more * details. * * You should have received a copy of the GNU General Public License along with * this program. If not, see <http://www.gnu.org/licenses/>. */ package com.l2jserver.gameserver.model.entity; import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.util.logging.Logger; import javolution.util.FastMap; import com.l2jserver.Config; import com.l2jserver.L2DatabaseFactory; import com.l2jserver.gameserver.ThreadPoolManager; import com.l2jserver.gameserver.datatables.CharNameTable; import com.l2jserver.gameserver.model.L2World; import com.l2jserver.gameserver.model.actor.instance.L2PcInstance; /** * @author Setekh */ public class Hitman { private static Hitman _instance; private FastMap <Integer, PlayerToAssasinate> _targets; private Logger _log = Logger.getLogger(Hitman.class.getName()); // Data Strings private static String SQL_SELECT = "select targetId,clientId,target_name,bounty,pending_delete from hitman_list"; private static String SQL_DELETE = "delete from hitman_list where targetId=?"; private static String SQL_SAVEING = "replace into `hitman_list` VALUES (?, ?, ?, ?, ?)"; private static String[] SQL_OFFLINE = { "select * from characters where char_name=?", "select * from characters where charId=?" }; // Clean every 15 mins ^^ private int MIN_MAX_CLEAN_RATE = 15 * 60000; // Fancy lookin public static boolean start() { if(Config.ALLOW_HITMAN_GDE) getInstance(); return _instance != null; } public static Hitman getInstance() { if(_instance == null) _instance = new Hitman(); return _instance; } public Hitman() { _targets = load(); ThreadPoolManager.getInstance().scheduleAiAtFixedRate(new AISystem(), MIN_MAX_CLEAN_RATE, MIN_MAX_CLEAN_RATE); } private FastMap<Integer, PlayerToAssasinate> load() { FastMap<Integer, PlayerToAssasinate> map = new FastMap<Integer, PlayerToAssasinate>(); try { Connection con = L2DatabaseFactory.getInstance().getConnection(); PreparedStatement st = con.prepareStatement(SQL_SELECT); ResultSet rs = st.executeQuery(); while(rs.next()) { int targetId = rs.getInt("targetId"); int clientId = rs.getInt("clientId"); String target_name = rs.getString("target_name"); int bounty = rs.getInt("bounty"); boolean pending = rs.getInt("pending_delete") == 1; if(pending) removeTarget(targetId, false); else map.put(targetId, new PlayerToAssasinate(targetId, clientId, bounty, target_name)); } _log.info("Hitman: Loaded "+map.size()+" Assassination Target(s)"); rs.close(); st.close(); con.close(); } catch(Exception e) { _log.warning("Hitman: "+e.getCause()); return new FastMap<Integer, PlayerToAssasinate>(); } return map; } public void onDeath(L2PcInstance assassin, L2PcInstance target) { if(_targets.containsKey(target.getObjectId())) { PlayerToAssasinate pta = _targets.get(target.getObjectId()); String name= getOfflineData(null, pta.getClientId())[1]; L2PcInstance client = L2World.getInstance().getPlayer(name); target.sendMessage("You have been assassinated. Your bounty is 0."); if(client != null) { client.sendMessage("Your assassination request to kill "+target.getName()+" has been fulfilled."); client.setHitmanTarget(0); } assassin.sendMessage("You assassinated "+target.getName()+", his bounty will be converted in adena!"); assassin.addAdena("Hitman", pta.getBounty(), target, true); removeTarget(pta.getObjectId(), true); } } public void onEnterWorld(L2PcInstance activeChar) { if(_targets.containsKey(activeChar.getObjectId())) { PlayerToAssasinate pta = _targets.get(activeChar.getObjectId()); activeChar.sendMessage("There is a hit on you. Worth " + pta.getBounty() + " adena(s)."); } if(activeChar.getHitmanTarget() > 0) { if(!_targets.containsKey(activeChar.getHitmanTarget())) { activeChar.sendMessage("Your target has been eliminated. Have a nice day."); activeChar.setHitmanTarget(0); } else activeChar.sendMessage("Your target is still at large."); } } public void save() { try { for(PlayerToAssasinate pta : _targets.values()) { Connection con = L2DatabaseFactory.getInstance().getConnection(); PreparedStatement st = con.prepareStatement(SQL_SAVEING); st.setInt(1, pta.getObjectId()); st.setInt(2, pta.getClientId()); st.setString(3, pta.getName()); st.setInt(4, pta.getBounty()); st.setInt(5, pta.isPendingDelete() ? 1 : 0); st.executeQuery(); st.close(); con.close(); } } catch(Exception e) { _log.warning("Hitman: "+e); } System.out.println("Hitman: List Saved."); } public void putHitOn(L2PcInstance client, String playerName, int bounty) { L2PcInstance player = L2World.getInstance().getPlayer(playerName); if(client.getHitmanTarget() > 0) { client.sendMessage("You are already a client here, you can place a request only for a single player."); return; } else if(bounty <= 100 ) { client.sendMessage("For a contract you must give more then 100 adena's."); return; } else if(client.getAdena() < bounty) { client.sendMessage("Not enough adena."); return; } else if(player == null && CharNameTable.getInstance().doesCharNameExist(playerName)) { Integer targetId = Integer.parseInt(getOfflineData(playerName, 0)[0]); if(_targets.containsKey(targetId)) { client.sendMessage("There is already a hit on that player."); return; } _targets.put(targetId, new PlayerToAssasinate(targetId, client.getObjectId(), bounty, playerName)); client.reduceAdena("Hitman", bounty, client, true); client.setHitmanTarget(targetId); } else if(player != null && CharNameTable.getInstance().doesCharNameExist(playerName)) { if(_targets.containsKey(player.getObjectId())) { client.sendMessage("There is already a hit on that player."); return; } player.sendMessage("There is a hit on you. Worth " + bounty + " Adena(s)."); _targets.put(player.getObjectId(), new PlayerToAssasinate(player, client.getObjectId(), bounty)); client.reduceAdena("Hitman", bounty, client, true); client.setHitmanTarget(player.getObjectId()); } else client.sendMessage("Player name invalid. The user u added dose not exist."); } public class AISystem implements Runnable { @Override public void run() { if(Config.DEBUG) _log.info("Cleaning sequance initiated."); for(PlayerToAssasinate target : _targets.values()) { if(target.isPendingDelete()) removeTarget(target.getObjectId(), true); } save(); } } public void removeTarget(int obId, boolean live) { try { Connection con = L2DatabaseFactory.getInstance().getConnection(); PreparedStatement st = con.prepareStatement(SQL_DELETE); st.setInt(1, obId); st.execute(); st.close(); con.close(); if(live) _targets.remove(obId); } catch(Exception e) { _log.warning("Hitman: "+e); } } public void cancelAssasination(String name, L2PcInstance client) { L2PcInstance target = L2World.getInstance().getPlayer(name); if(client.getHitmanTarget() <= 0) { client.sendMessage("You don't own a hit."); return; } else if(target == null && CharNameTable.getInstance().doesCharNameExist(name)) { PlayerToAssasinate pta = _targets.get(client.getHitmanTarget()); if(!_targets.containsKey(pta.getObjectId())) client.sendMessage("There is no hit on that player."); else if(pta.getClientId() == client.getObjectId()) { removeTarget(pta.getObjectId(), true); client.sendMessage("The hit has been canceld."); client.setHitmanTarget(0); } else client.sendMessage("You are not the actual owner of that target!."); } else if(target != null && CharNameTable.getInstance().doesCharNameExist(name)) { PlayerToAssasinate pta = _targets.get(target.getObjectId()); if(!_targets.containsKey(pta.getObjectId())) client.sendMessage("There is no hit on that player."); else if(pta.getClientId() == client.getObjectId()) { removeTarget(pta.getObjectId(), true); client.sendMessage("The hit has been canceld."); target.sendMessage("The hit on you has been canceld."); client.setHitmanTarget(0); } else client.sendMessage("You are not the actual owner of that target!."); } else client.sendMessage("Player name invalid. The user u added dose not exist."); } /** * Its useing a array in case in a future update more values will be added * @param name */ public String[] getOfflineData(String name, int objId) { String[] set = new String[2]; try { Connection con = L2DatabaseFactory.getInstance().getConnection(); PreparedStatement st = con.prepareStatement(objId > 0 ? SQL_OFFLINE[1] : SQL_OFFLINE[0]); if(objId > 0) st.setInt(1, objId); else st.setString(1, name); ResultSet rs = st.executeQuery(); while(rs.next()) { set[0] = String.valueOf(rs.getInt("charId")); set[1] = rs.getString("char_name"); } rs.close(); st.close(); con.close(); } catch(Exception e){ _log.warning("Hitman: "+e); } return set; } public boolean exists(int objId) { return _targets.containsKey(objId); } public PlayerToAssasinate getTarget(int objId) { return _targets.get(objId); } /** * @return the _targets */ public FastMap<Integer, PlayerToAssasinate> getTargets() { return _targets; } /** * @param targets the _targets to set */ public void set_targets(FastMap<Integer, PlayerToAssasinate> targets) { _targets = targets; } } -
hello, i have an hitman system but i don't know how to change the reward item it looks like assassin.addAdena("Hitman", pta.getBounty(), target, true); i rlly need it :) edit: fixed ^^
-
[help] vote reward bugged
TouchAndDie replied to TouchAndDie's question in Request Server Development Help [L2J]
up :'( :'( -
[help] vote reward bugged
TouchAndDie replied to TouchAndDie's question in Request Server Development Help [L2J]
up -
hello :) i have this vote reward: package com.l2jserver.gameserver.instancemanager; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.net.URL; import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import com.l2jserver.L2DatabaseFactory; import com.l2jserver.gameserver.Announcements; import com.l2jserver.gameserver.ThreadPoolManager; import com.l2jserver.gameserver.model.L2ItemInstance; import com.l2jserver.gameserver.model.L2World; import com.l2jserver.gameserver.model.actor.instance.L2PcInstance; import com.l2jserver.gameserver.network.clientpackets.Say2; import com.l2jserver.gameserver.network.serverpackets.CreatureSay; import com.l2jserver.gameserver.util.Broadcast; public class AutoVoteRewardHandler { private final String TOPZONE = "http://l2topzone.com/"; // 60 * 1000(1000milliseconds = 1 second) = 60seconds private final int initialCheck = 60 * 1000; // 1800 * 1000(1000milliseconds = 1 second) = 1800seconds = 30minutes private final int delayForCheck = 1800 * 1000; private final int[] itemId = { 9627, 9142 }; private final int[] itemCount = { 2, 500 }; private final int[] maxStack = { 2, 500 }; private final int votesRequiredForReward = 10; // do not change private int lastVoteCount = 0; private AutoVoteRewardHandler() { System.out.println("Vote Reward System Initiated."); ThreadPoolManager.getInstance().scheduleGeneralAtFixedRate(new AutoReward(), initialCheck, delayForCheck); } private class AutoReward implements Runnable { public void run() { int votes = getVotes(); System.out.println("Server Votes: " + votes); if (votes != 0 && getLastVoteCount() != 0 && votes >= getLastVoteCount() + votesRequiredForReward) { Connection con = null; try { con = L2DatabaseFactory.getInstance().getConnection(); PreparedStatement statement = con.prepareStatement("SELECT c.charId, c.char_name FROM characters AS c LEFT JOIN accounts AS a ON c.account_name = a.login WHERE c.online > 0 GROUP BY a.lastIP ORDER BY c.level DESC"); ResultSet rset = statement.executeQuery(); L2PcInstance player = null; L2ItemInstance item = null; while (rset.next()) { player = L2World.getInstance().getPlayer(rset.getInt("charId")); if (player != null && !player.getClient().isDetached()) { for (int i = 0; i < itemId.length; i++) { item = player.getInventory().getItemByItemId(itemId[i]); if (item == null || item.getCount() < maxStack[i]) player.addItem("reward", itemId[i], itemCount[i], player, true); } } } statement.close(); } catch (SQLException e) { e.printStackTrace(); } finally { L2DatabaseFactory.close(con); } setLastVoteCount(getLastVoteCount() + votesRequiredForReward); } Broadcast.toAllOnlinePlayers(new CreatureSay(1, Say2.ANNOUNCEMENT, "Announcements:", "Server Votes: " + votes + " | Next Reward on " + (getLastVoteCount() + votesRequiredForReward) + " Votes.)")); if (getLastVoteCount() == 0) setLastVoteCount(votes); } } private int getVotes() { URL url = null; InputStreamReader isr = null; BufferedReader in = null; try { url = new URL(TOPZONE); isr = new InputStreamReader(url.openStream()); in = new BufferedReader(isr); String inputLine; while ((inputLine = in.readLine()) != null) { if (inputLine.contains("rank anonymous tooltip")) { return Integer.valueOf(inputLine.split(">")[2].replace("</span", "")); } } } catch (IOException e) { e.printStackTrace(); } finally { try { in.close(); } catch (IOException e) {} try { isr.close(); } catch (IOException e) {} } return 0; } private void setLastVoteCount(int voteCount) { lastVoteCount = voteCount; } private int getLastVoteCount() { return lastVoteCount; } public static AutoVoteRewardHandler getInstance() { return SingletonHolder._instance; } @SuppressWarnings("synthetic-access") private static class SingletonHolder { protected static final AutoVoteRewardHandler _instance = new AutoVoteRewardHandler(); } } if the script check it and it has 10 votes i get vote reward but if the check is with 11 votes+ i don't get the reward.. i mean now i have 100 votes and after 2 mins i have 111 votes and i don't get the reward only if i have 110.. how can i fix that ?
-
how can i put skill enchant limit to +15 ?
-
Help for install rin4a buffer please.
TouchAndDie replied to Zenith's question in Request Server Development Help [L2J]
check in data/scripts/custom/buffer.py this: NPC_ID = if is your NPC ID :) if not put there the npc id :) -
i have a clan rep manager but i don't know how to change the items requied for reputation :) action="bypass -h npc_%objectId%_exchange 1591" finded :D ( l2ClanTraderInstance.java )
-
i have a gh, i am full, vorpal+16,rb jwls+16, etc... i have 4.9k p def and 4.6k m def and another player have same gear like mine but he have 6.5k pdef and 6.2k m def :-? anyone know what's the trick ?
-
Lineage 2 Pavilion High Five PVP Server!
TouchAndDie replied to BloOdDiamOnD's topic in Private Servers
really nice server but they need more players :( cmon join us :D -
hmm where are the npc's ? :D btw do you know how to develop ? and you are using arenagames system ^^
-
[help] shout timer
TouchAndDie replied to TouchAndDie's question in Request Server Development Help [L2J]
thank you. -
[help] shout timer
TouchAndDie replied to TouchAndDie's question in Request Server Development Help [L2J]
oops :D how can i change the message "Do not spam on global chat" ? -
[help] shout timer
TouchAndDie replied to TouchAndDie's question in Request Server Development Help [L2J]
its 250 .. but don't work -
[help] shout timer
TouchAndDie replied to TouchAndDie's question in Request Server Development Help [L2J]
i could not find it in source :| -
How can i put a timer on Shout ? i mean after someone wrote somthing on Shout ( ! ) he cannot write again 20 sec:D
-
WTB [WTB] Goddess of Destruction server
TouchAndDie replied to TouchAndDie's topic in Marketplace [L2Packs & Files]
no :)) -
WTB Goddess of Destruction java source with 4th Classes, Full skills, Full items, Full spawnlist, etc. PM Me !
-
[req] god source
TouchAndDie replied to TouchAndDie's question in Request Server Development Help [L2J]
:D -
[req] god source
TouchAndDie replied to TouchAndDie's question in Request Server Development Help [L2J]
:-s Remind your players to DONT use the new NC Launcher to run the game, if they update their client they will be not able to log into your server. when i wanna run the game i get critical error.. History: FFileManagerWindows::CreateFileReader <- appLoadFileToString <- FConfigFile::Read <- FConfigCacheIni::Find <- FConfigCacheIni::GetString <- UObject::LoadConfig <- (PlayerController None) <- UClass::Serialize <- (Class Engine.PlayerController) <- LoadObject <- (Class Engine.PlayerController 155626==155626/2806942 148528 7098) <- ULinkerLoad::Preload <- PreLoadObjects <- UObject::EndLoad <- UObject::StaticLoadObject <- (Core.Class Engine.erty Engine.PlayerController.ClientValidate.C NULL) <- UObject::StaticLoadClass <- InitEngine i've updated god with NC Launcher, that's the problem ? if yes, where i can find GoD English client ? -
[req] god source
TouchAndDie replied to TouchAndDie's question in Request Server Development Help [L2J]
Thank you ! ;D -
[req] god source
TouchAndDie replied to TouchAndDie's question in Request Server Development Help [L2J]
if you tell me how, it will be wonderfull :D