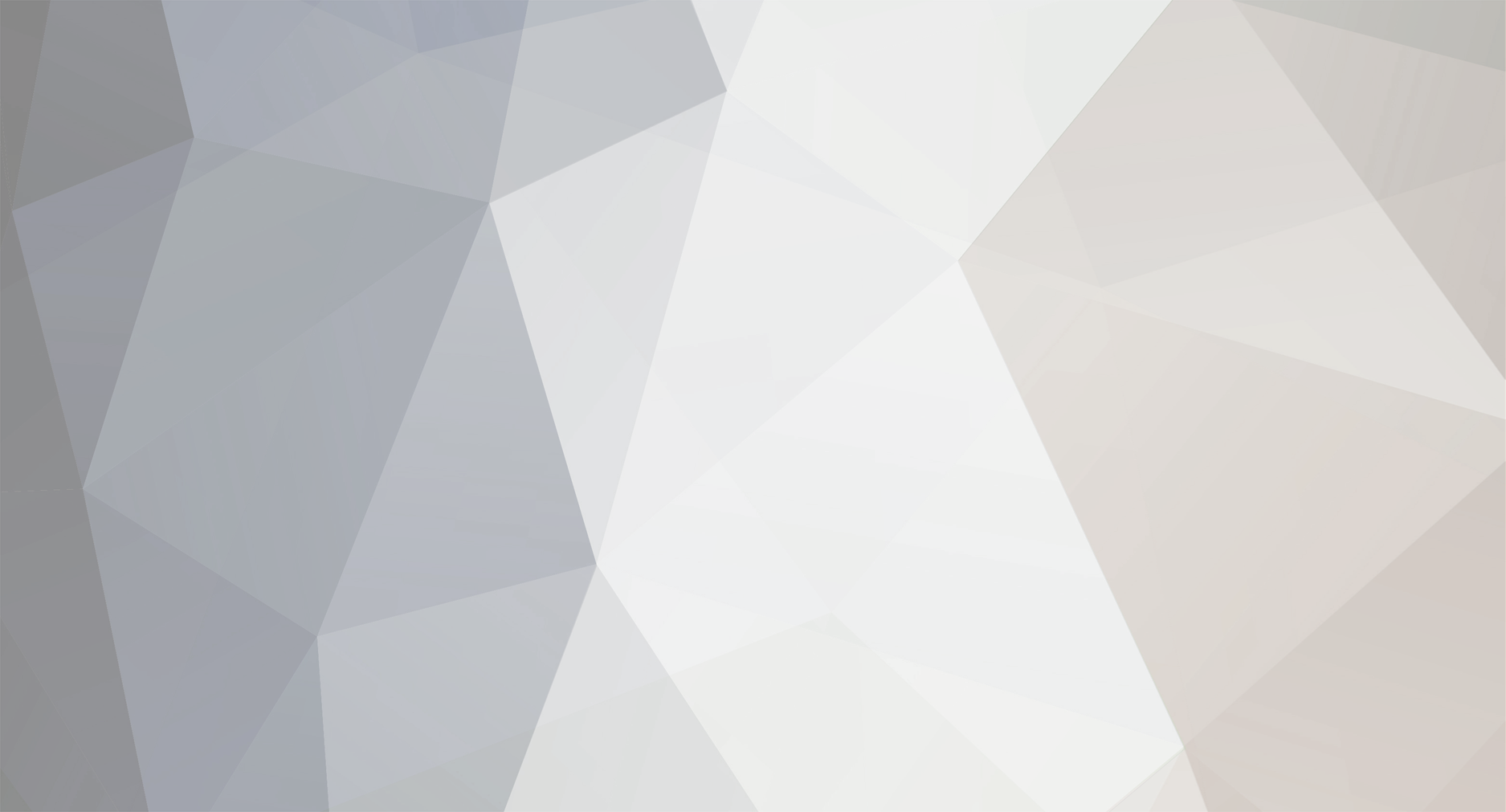
Inthedash6
-
Posts
81 -
Credits
0 -
Joined
-
Last visited
-
Feedback
0%
Content Type
Articles
Profiles
Forums
Store
Posts posted by Inthedash6
-
-
Hi everyone,sell l2j.lt top 10. more info skype: intheend15
-
Code:
/* * This program is free software; you can redistribute it and/or modify * it under the terms of the GNU General Public License as published by * the Free Software Foundation; either version 2, or (at your option) * any later version. * * This program is distributed in the hope that it will be useful, * but WITHOUT ANY WARRANTY; without even the implied warranty of * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * GNU General Public License for more details. * * You should have received a copy of the GNU General Public License * along with this program; if not, write to the Free Software * Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA * 02111-1307, USA. * * http://www.gnu.org/copyleft/gpl.html */ package com.l2jfrozen.gameserver.model.actor.instance; import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.util.StringTokenizer; import java.util.logging.Level; import com.l2jfrozen.util.database.L2DatabaseFactory; import com.l2jfrozen.gameserver.thread.ThreadPoolManager; import com.l2jfrozen.gameserver.cache.HtmCache; import com.l2jfrozen.gameserver.templates.L2NpcTemplate; import com.l2jfrozen.gameserver.network.serverpackets.NpcHtmlMessage; public class L2StatusInstance extends L2NpcInstance { private class PlayerInfo { public PlayerInfo(int pos,String n, int pvps, int pks ,int ontime, Boolean iso) { position = pos; Nick = n; pvpCount = pvps; pkCount = pks; onlineTime = ontime; isOnline = iso; } public int position; public String Nick; public int pvpCount; public int pkCount; public int onlineTime; public Boolean isOnline; } //delay interval (in minutes): private final int delayForCheck = 5; //number of players to be listed private int pvpListCount = 10; private int pkListCount = 10; private int onlineListCount = 10; private PlayerInfo [] topPvPList = new PlayerInfo [pvpListCount]; private PlayerInfo [] topPkList = new PlayerInfo [pkListCount]; private PlayerInfo [] topOnlineList = new PlayerInfo [onlineListCount]; public L2StatusInstance(int objectId, L2NpcTemplate template) { super(objectId, template); ThreadPoolManager.getInstance().scheduleGeneralAtFixedRate(new RefreshAllLists(), 10000, delayForCheck * 60000); } private class RefreshAllLists implements Runnable { public void run() { ReloadData(); } } private void ReloadData() { try (Connection con = L2DatabaseFactory.getInstance().getConnection()) { PreparedStatement statement = con.prepareStatement("SELECT char_name, pvpkills, online FROM characters ORDER BY pvpkills DESC, char_name ASC LIMIT 10"); ResultSet result = statement.executeQuery(); //refreshing top pvp list int i = 0; //index of array while (result.next()) { topPvPList[i] = new PlayerInfo(i+1,result.getString("char_name"),result.getInt("pvpkills"),0,0,result.getBoolean("online")); i++; } //refreshing top pk list statement = con.prepareStatement("SELECT char_name, pkkills, online FROM characters ORDER BY pkkills DESC, char_name ASC LIMIT 10"); result = statement.executeQuery(); i = 0; //index of array while (result.next()) { topPkList[i] = new PlayerInfo(i+1,result.getString("char_name"),0,result.getInt("pkkills"),0,result.getBoolean("online")); i++; } //refreshing top online list statement = con.prepareStatement("SELECT char_name, onlinetime, online FROM characters ORDER BY onlinetime DESC, char_name ASC LIMIT 10"); result = statement.executeQuery(); i = 0; //index of array while (result.next()) { topOnlineList[i] = new PlayerInfo(i+1,result.getString("char_name"),0,0,result.getInt("onlinetime"),result.getBoolean("online")); i++; } result.close(); statement.close(); } catch (SQLException e) { _log.log(Level.WARNING, "ranking (status): could not load statistics informations" + e.getMessage(), e); } } @Override public void onSpawn() { ReloadData(); } @Override public void showChatWindow(L2PcInstance player) { GeneratePvPList(player); } @Override public void onBypassFeedback(L2PcInstance player, String command) { StringTokenizer st = new StringTokenizer(command, " "); String currentCommand = st.nextToken(); if (currentCommand.startsWith("pvplist")) { GeneratePvPList(player); } else if (currentCommand.startsWith("pklist")) { GeneratePKList(player); } else if (currentCommand.startsWith("onlinelist")) { GenerateOnlineList(player); } super.onBypassFeedback(player, command); } private void GeneratePvPList(L2PcInstance p) { StringBuilder _PVPranking = new StringBuilder(); for (PlayerInfo player : topPvPList) { if (player == null) break; _PVPranking.append("<table width=\"290\"><tr>"); _PVPranking.append("<td FIXWIDTH=\"2\" align=\"center\"></td>"); _PVPranking.append("<td FIXWIDTH=\"17\" align=\"center\">"+player.position+"</td>"); _PVPranking.append("<td FIXWIDTH=\"158\" align=\"center\">"+player.Nick+"</td>"); _PVPranking.append("<td FIXWIDTH=\"90\" align=\"center\">"+player.pvpCount+"</td>"); _PVPranking.append("<td FIXWIDTH=\"50\" align=\"center\">"+((player.isOnline) ? "<font color=\"00FF00\">ON</font>" : "<font color=\"CC0000\">OFF</font>")+"</td>"); _PVPranking.append("<td FIXWIDTH=\"2\" align=\"center\"></td>"); _PVPranking.append("</tr></table>"); _PVPranking.append("<img src=\"L2UI.Squaregray\" width=\"300\" height=\"1\">"); } NpcHtmlMessage html = new NpcHtmlMessage(1); html.setFile(getHtmlPath(getNpcId(), 0)); html.replace("%objectId%", getObjectId()); html.replace("%pvplist%", _PVPranking.toString()); p.sendPacket(html); } private void GeneratePKList(L2PcInstance p) { StringBuilder _PVPranking = new StringBuilder(); for (PlayerInfo player : topPkList) { if (player == null) break; _PVPranking.append("<table width=\"290\"><tr>"); _PVPranking.append("<td FIXWIDTH=\"2\" align=\"center\"></td>"); _PVPranking.append("<td FIXWIDTH=\"17\" align=\"center\">"+player.position+"</td>"); _PVPranking.append("<td FIXWIDTH=\"158\" align=\"center\">"+player.Nick+"</td>"); _PVPranking.append("<td FIXWIDTH=\"90\" align=\"center\">"+player.pkCount+"</td>"); _PVPranking.append("<td FIXWIDTH=\"50\" align=\"center\">"+((player.isOnline) ? "<font color=\"00FF00\">ON</font>" : "<font color=\"CC0000\">OFF</font>")+"</td>"); _PVPranking.append("<td FIXWIDTH=\"2\" align=\"center\"></td>"); _PVPranking.append("</tr></table>"); _PVPranking.append("<img src=\"L2UI.Squaregray\" width=\"300\" height=\"1\">"); } NpcHtmlMessage html = new NpcHtmlMessage(1); html.setFile(getHtmlPath(getNpcId(), 2)); html.replace("%objectId%", getObjectId()); html.replace("%pklist%", _PVPranking.toString()); p.sendPacket(html); } private void GenerateOnlineList(L2PcInstance p) { StringBuilder _PVPranking = new StringBuilder(); for (PlayerInfo player : topOnlineList) { if (player == null) break; _PVPranking.append("<table width=\"290\"><tr>"); _PVPranking.append("<td FIXWIDTH=\"2\" align=\"center\"></td>"); _PVPranking.append("<td FIXWIDTH=\"17\" align=\"center\">"+player.position+"</td>"); _PVPranking.append("<td FIXWIDTH=\"158\" align=\"center\">"+player.Nick+"</td>"); _PVPranking.append("<td FIXWIDTH=\"90\" align=\"center\">"+ConverTime(player.onlineTime)+"</td>"); _PVPranking.append("<td FIXWIDTH=\"50\" align=\"center\">"+((player.isOnline) ? "<font color=\"00FF00\">ON</font>" : "<font color=\"CC0000\">OFF</font>")+"</td>"); _PVPranking.append("<td FIXWIDTH=\"2\" align=\"center\"></td>"); _PVPranking.append("</tr></table>"); _PVPranking.append("<img src=\"L2UI.Squaregray\" width=\"300\" height=\"1\">"); } NpcHtmlMessage html = new NpcHtmlMessage(1); html.setFile(getHtmlPath(getNpcId(), 3)); html.replace("%objectId%", getObjectId()); html.replace("%onlinelist%", _PVPranking.toString()); p.sendPacket(html); } private String ConverTime(long seconds) { long remainder = seconds; int days = (int) remainder / (24*3600); remainder = remainder -(days * 3600 * 24); int hours = (int) (remainder / 3600); remainder = remainder -(hours * 3600); int minutes = (int) (remainder / 60); remainder = remainder -(hours * 60); seconds = remainder; String timeInText = ""; if (days > 0) timeInText = days+"<font color=\"LEVEL\">D</font> "; if (hours > 0) timeInText = timeInText+ hours+"<font color=\"LEVEL\">H</font> "; if (minutes >0) timeInText = timeInText+ minutes+"<font color=\"LEVEL\">M</font>"; if (timeInText=="") { if(seconds>0) { timeInText = seconds+"<font color=\"LEVEL\">S</font>"; } else { timeInText = "N/A"; } } return timeInText; } @Override public String getHtmlPath(int npcId, int val) { String filename; if (val == 0) filename = "data/html/Status/" + npcId + ".htm"; else filename = "data/html/Status/" + npcId + "-" + val + ".htm"; if (HtmCache.getInstance().isLoadable(filename)) return filename; return "data/html/Status/" + npcId + ".htm"; } }
-
I have error (Frozen)
NpcHtmlMessage html = new NpcHtmlMessage(1);html.setFile(getHtmlPath(getNpcId(), 0));html.replace("%objectId%", getObjectId());html.replace("%pvplist%", _PVPranking.toString());p.sendPacket(html);}Need help -
Server start tomorrow. (04-25) 17:00 GMT+2
-
Up up up
-
Hello dear players,i would like to introduce you our new server. Start Tommorow 2015.04.15 17:00 GMT+2
Server RatesXP: 999xSP: 999xAdena: 999xDropA: 1xEnchantEnchant Max Normal/Blessed: +15Enchant Max Crystal: +19Enchant Safe: +3Simple Enchant Rates: 65%Bessed Enchant Rates: 85%Crystal Enchant Rates: 100%Custom ItemsVesper Super ArmorsTriumph WeaponEpic WingsTattooBasic ThingsClient - Interlude.Main town - Giran.Castle crown witch stat.Wedding system.Max buff slot - 60.Max SubClass - 3.Spawn potection 30s.RaidBoss 8.Geodata.Quake system.Auto Vote Reward System.Destroy SS/BSS.Auto TvT,CTF Event.Clan rep item (5000 rep).Leave buff after death.Nick color: 100,300,500,700,1000 PvP.Title color: 50,100,200,350,500 PK.10 PvP- Hero aura.Anti Heavy System.Custom Items.GM shop.Augmentation(15%).Duel systema.Interlude skill (90%).Sub classes without quest.Nobless without quest.Npc Buffer.Buff time 9 hours.CommandTvt: .jointvt and .leavetvtOnline: .onlineHeal: .buffsServer NPCGM ShopCustom ShopNPC BufferName ChangerAugmenter/Skill EnchanterRaidBoss ManagerBug ReporterGateKeeperPK ProtectorAnd more...OlympiadStart - 18:00 (GMT +2)End - 00:00 (GMT +2)Olympiad only without custom Items.Olympiad system Every 1 Weeks (Full Working), Retail OlympiadHero Change Every Friday Thursday 16:00Validation period 12h
-
Server back. Today (03-07) 17:00GMT+2.
Rates:
Exp - 2000x/ Sp - 2000x/ Aden - 2000x/ Drop - 1x
Enchant rates:
Enchant Max Weapon: +25
Enchant Max Armor/Jewels: +30
Enchant Safe: +5
Simple Enchant Rates: 70%
Bessed Enchant Rates: 95%
Other:
# Client - Interlude.
# Main town - Giran.
# Castle crown witch stat.
# Wedding system.
# Max buff slot - 80.
# Max SubClass - 5.
# Spawn potection 20s.
# RaidBoss 5.
# Geodata.
# Quake system.
# Auto Vote Reward System.
# Don't Destroy SS/BSS.
# Auto TvT,CTF Event.
# Auto server restart 03:00.
# Clan rep item.
# Don't leave buff after death.
# Nick color: 100,300,500,700,1000 PvP.
# Title color: 50,100,200,350,500 PK.
# 10 PvP- Hero aura.
# Anti Heavy System.
# Custom Items.
# GM shop.
# Pc Bang System.
# Augmentation(11%).
# Duel systema.
# Interlude skill (90%).
# Sub classes without quest.
# Nobless without quest.
# Npc Buffer.
# Server have 8 achievements.
# Buff time 2 hours.
Custom Item:.
# Armor: Vesper Blue.
# Weapon: Vesper Noble.
# Aksesuars: Wings 1/2 Lv.
# Other: Tatto witch stat. 1/2 Lv
Olympiad:.
# Period: 1 Week.
# Start 18:00h GMT+2.
# End 00:00h GMT+2.
# Hero change every Friday 23:01.
# After match players teleport to town.
Command:.
# .tvtjoin/.tvtleave - Join or Leave TvT Event.
# .ctfjoin/.ctfleave - Join or Leave CTF Event.
# .repair - Fix charachter.
# .pvpinfo - Can see you pvp rank.
# .menu - See how much players are online and off/on your Trade/PM.
# .rewardme - For 5 Event item you have Super Haste skill 1Lvl for 15minutes. -
http://l2fusion.ql.lt/ This friday 03.07 17:00 GMT+2
-
Fix link,any have code?
-
uppppp
-
upp
-
Start Today 18:00 GMT+2
-
upppppppppp
-
Uppppppp
-
Hello everyone, you remember old L2 Fusion? Now he converted into a custom server. I want to let you know that the L2Fusion Custom PvP x2000 server startsThis Friday 03.07.2014 17:00 GMT +2 Time). In server we worked, not really short time and we hope that will be no errors. So, we waiting for you in our server and let the new battle begin! Invite your friends, and you know that more players its more interesting!! .Soon we will get the domain.
Rates:
Exp - 2000x/ Sp - 2000x/ Aden - 2000x/ Drop - 1x
Enchant rates:
Enchant Max Weapon: +25
Enchant Max Armor/Jewels: +30
Enchant Safe: +5
Simple Enchant Rates: 70%
Bessed Enchant Rates: 95%
Other:
# Client - Interlude.
# Main town - Giran.
# Castle crown witch stat.
# Wedding system.
# Max buff slot - 80.
# Max SubClass - 5.
# Spawn potection 20s.
# RaidBoss 5.
# Geodata.
# Quake system.
# Auto Vote Reward System.
# Don't Destroy SS/BSS.
# Auto TvT,CTF Event.
# Auto server restart 03:00.
# Clan rep item.
# Don't leave buff after death.
# Nick color: 100,300,500,700,1000 PvP.
# Title color: 50,100,200,350,500 PK.
# 10 PvP- Hero aura.
# Anti Heavy System.
# Custom Items.
# GM shop.
# Pc Bang System.
# Augmentation(11%).
# Duel systema.
# Interlude skill (90%).
# Sub classes without quest.
# Nobless without quest.
# Npc Buffer.
# Server have 8 achievements.
# Buff time 2 hours.
Custom Item:.
# Armor: Vesper Blue.
# Weapon: Vesper Noble.
# Aksesuars: Wings 1/2 Lv.
# Other: Tatto witch stat. 1/2 Lv
Olympiad:.
# Period: 1 Week.
# Start 18:00h GMT+2.
# End 00:00h GMT+2.
# Hero change every Friday 23:01.
# After match players teleport to town.
Command:.
# .tvtjoin/.tvtleave - Join or Leave TvT Event.
# .ctfjoin/.ctfleave - Join or Leave CTF Event.
# .repair - Fix charachter.
# .menu - See how much players are online and off/on your Trade/PM.
# .rewardme - For 5 Event item you have Super Haste skill 1Lvl for 15minutes. -
-
-
-
-
We are glad to present you our server, much more active and improved than ever! We are currently working too hard to make server balanced and enjoyable!
Server don't have any custom items or tattoo.
Server start Friday 17:00 GMT+2 Our system was uploaded 2 hours before start.See you in game.
http://l2chaoslegion.eu/
Features
Rates:
» Xp 1000x.
» Sp 1000x.
» Aden 1000x.
» Drop 1x.
» Starting character level - 39.
Enchant rates:
» Safe enchant +2.
» Blessed and simple scrolls max enchant (+7).
» Crystal scrolls max enchant (+10).
» Simple enchant scrolls chance - 60%.
» Blessed enchant scrolls chance - 70%.
» Crystal enchant scrolls chance - 90%.
Unique features:
» No Custom items!
» No Tattoo!
» Apella Armor!
» Rb Jewels from GrandBoss or Special Shop.
» Main town - Giran.
» Castle sieges.
» Wedding system.
» Unique farming zones.
» Npc skill enchanter.
» A grade items for free.
» Shops till top S grade.
» Npc buffer.
» Max count of buffs - 42.
» Max subclasses - 3.
» Free and no quest class change.
» Free and no quest sub class.
» No nobless quest.
» No weight limit.
» No grade limit.
» Bug report NPC.
» Auto server restart 01:00.
» Quake pvp system.
» War Legend Aura - 10PvP.
» Nick/Title color system.
» Achievements system.
» Online password change.
» Top 20 pvp/pk npc in game.
» Augmentation system (1 Passive+1 Active).
» Server information npc.
» Unique monsters.
» Interlude retail skills.
» Server up-time [24/7] [99]%.
» Perfect class balance.
Voiced commands.
.tvtjoin .tvtleave - Join or leave tvt event.
.ctfjoin .ctfleave - Join or leave ctf event.
.online - current online players count.
.repair - repairs stuck character in world.
Event system:
» TVT event [12:00 15:00 17:00 20:00 23:00] hours.
» CTF event [13:00 16:00 19:00 21:00 24:00] hours.
Olympiad game:
» Retail olympiad game.
» Competition period [1] week.
» Olympiad start time [18:00] end [23:00]. -
Start today 18:00 gmt+3 http://l2away.eu/informacija.php
-
But for me working. I don't know,why don't work for u...
-
Invite event: http://l2away.eu/invite/
-
up
Clan Memeber
in Request Server Development Help [L2J]
Posted
Hello,how I can change clan member limit? and how much need to clan level up rep? Sorry for bad english.