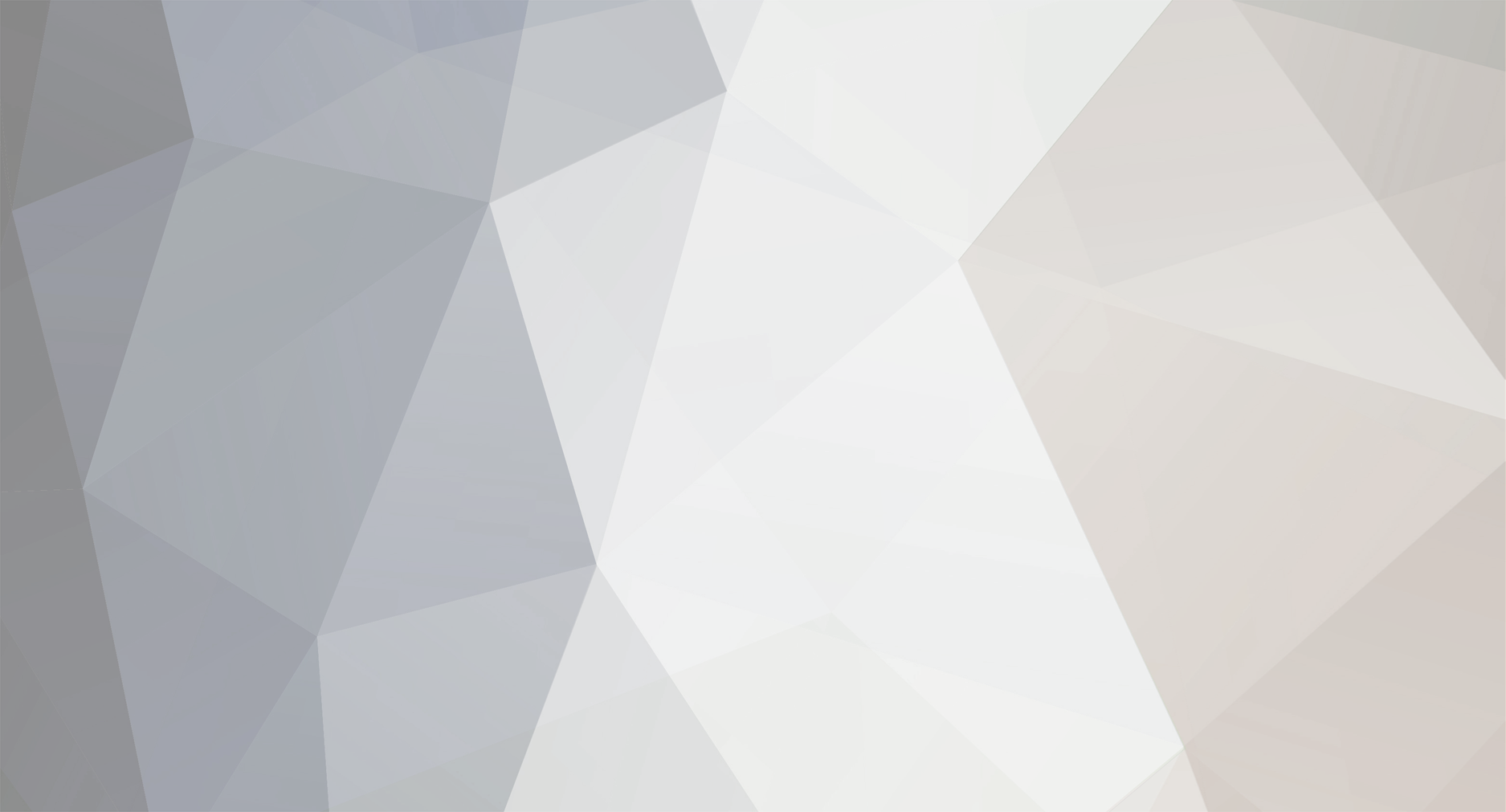
alexak0s1
-
Posts
130 -
Credits
0 -
Joined
-
Last visited
-
Feedback
0%
Content Type
Articles
Profiles
Forums
Store
Posts posted by alexak0s1
-
-
solved im an idiot :D lock this ... ( all files are acis gameserver /build / dist )
-
i did that with eclipse on build xml with ant 1 :P but there werent any zip sorry im new :(
-
After using eclipse i used database installer , everything went ok but when i tried to register the gameserver i got this error !
Sorry for my english !
thanks.
-
if you dont like it .. then dont play it .. Dont abuse
-
-
fail from what ?
-
Mages... enas filos m thelei na ani3ei server kai egw ton psilovoithaw oso borw..
O server einai freya!
Epidi theloume na lene ola ta class theloume na valoume blade rush ston blade dancer..
Ta skill tree ta exei mesa sto navicat .. id tou class tou spectral dancer dld 107 evala kai to id tou skill p einai 495 kai dn emfanizete tpt stous spectral dancers... pws borw na to ftia3w ?
Sas euxaristw polu esto p to diavasate :)!
-
i fixed itttt :forever alone like a boss: :-beep- yeah:
-
test our server .! :)
-
Game Specifications :
Server Chronicle:
Freya
Rates:
XP: x5000
SP: x5000
Adena: x2500
Enchant Rates:
Max: +25
Safe: +7
Chance: 70%
Blessed: 90%
Crystal: 100%
Event :
*TvT Event(Auto)-APIGA
*Death Match Event [GM]-Gold Dragon[hero for 24H]
*Hide and seek with gms - Items +25
*PvP Event At coliseum made by Gm Team* With prize 1 Active skill or +1 more on a +25 weapon (1 time per month)
*Russian rullete-Unique items(Monster shields, Coins and more...)
Vote Reward System :
We have a special event & vote reward system which is based on Apigas.!
With every 5 votes on Topzone, all online players will be automatically rewarded with Vote Reward Item.
General Informations:
Olympiad :
*Validation Period every 1 weeks.
*From 18:00 to 24:00 GMT +2.
*Hero weapons CAN NOT be enchanted.
Noblesse :
*Available Caradine's Letter at Gm Shop
Subclass :
- Up to 3 subclasses.
Custom Items:
Apiga.
Bloody Pa'agrio
Custom NPCs :
*Gmshop
*Global Gatekeeper
*Buffer
*Custom Shop
*Wedding Manager
*Class Changer
*Augmenter
*Title colour changer!*
*PvP/Pk List Manager)
Uptime : 100.0%
Our Site : http://l2dot.zxq.net/ Join us !
-
can i have a link ?
-
Can i have a buffer with full fighter buffs and mage buffs working on this pack ? becouse no script is working on my pack
l2jhellas
-
AGORINA M SAGAPAW someone lock it please :$
-
Pedia ekana compile ena l2jhellas pack kai stin database dn exei armorsets :rage: ti bwrw na kanw etsi oste na valw mia custom armor me armorsets ? :)
-
Kalispera :D
Exw 1 problima Me To Ally Creation Otan Patas Ally dn s dixnei tpt S leei Oti Xriazese quest Mipos boroume na to lisoume?
-
A OK FIXED TY :/ TO EIXA XEXASI :d
-
Exw 1 provlima me to hexid to pack einai l2 j archid kai dn m kanei register hexid pos alios kanw :/
-
:'(tha kanw copy paste i kati allo ? giati java code :/ Dn xerw XD
-
Kalispera
Exw Ena Problima Me Ta Subclass Kanei Mono 3 Sub Kai Thelw Na To Megaloso GinetE? Episis Dn M Kanei Cancel SubClass Pos To Ftiaxnw
[move]Thx :D[/move]
-
Kalispera
Thelw Na Mathw Pos Ginete Na Alaxw TO MAX Apo Ta Subclass Pou Bori Na Kanei O Kathenas Dioti Exw 1 Problima Dn Kanei Cansel Subclass >:( Opios Xeri Parakalo Na Me Voithisi :-*
-
60 ppl :)
-
40 ppl reached :)
-
You "WILL" buy eu site ... s00r ..
Don't Even think to call me "s_cker" again ...
Etc homemade come on ... 1 ppl online when i join .. me and you .. loled ... !!
i realy will
-
full guards?
they jerk it of more than the admins. i got pked 2 times at a -beep-ing safe zone
we will make safe zone safe zone i mean they wont hit you in that zone
Hopzone & Topzone Vote Reward System
in Adapted customs
Posted · Edited by alexak0s1
((((((((((((((((((well i have problem on Announcement.announceToAll the announcements path is moved and changed i found it and put it in but i still get this announcetoall error))))))))
/*
* This program is free software: you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License along with
* this program. If not, see <http://www.gnu.org/licenses/
.
*/
package net.sf.l2j.gameserver.model.entity;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.URL;
import java.net.URLConnection;
import java.util.Collection;
import java.util.HashMap;
import net.sf.l2j.Config;
import net.sf.l2j.gameserver.model.Announcement;
import net.sf.l2j.gameserver.ThreadPoolManager;
import net.sf.l2j.gameserver.model.L2World;
import net.sf.l2j.gameserver.model.actor.instance.L2PcInstance;
/**
* @author Anarchy
*
*/
public class VoteRewardTopzone
{
// Configurations.
private static String topzoneUrl = Config.TOPZONE_SERVER_LINK;
private static String page1Url = Config.TOPZONE_FIRST_PAGE_LINK;
private static int voteRewardVotesDifference = Config.TOPZONE_VOTES_DIFFERENCE;
private static int firstPageRankNeeded = Config.TOPZONE_FIRST_PAGE_RANK_NEEDED;
private static int checkTime = 60*1000*Config.TOPZONE_REWARD_CHECK_TIME;
// Don't-touch variables.
private static int lastVotes = 0;
private static HashMap<String, Integer> playerIps = new HashMap<>();
public static void updateConfigurations()
{
topzoneUrl = Config.TOPZONE_SERVER_LINK;
page1Url = Config.TOPZONE_FIRST_PAGE_LINK;
voteRewardVotesDifference = Config.TOPZONE_VOTES_DIFFERENCE;
firstPageRankNeeded = Config.TOPZONE_FIRST_PAGE_RANK_NEEDED;
checkTime = 60*1000*Config.TOPZONE_REWARD_CHECK_TIME;
}
public static void getInstance()
{
System.out.println("Topzone - Vote reward system initialized.");
ThreadPoolManager.getInstance().scheduleGeneralAtFixedRate(new Runnable()
{
@Override
public void run()
{
if (Config.ALLOW_TOPZONE_VOTE_REWARD)
{
reward();
}
else
{
return;
}
}
}, checkTime/2, checkTime);
}
static void reward()
{
int firstPageVotes = getFirstPageRankVotes();
int currentVotes = getVotes();
if (firstPageVotes == -1 || currentVotes == -1)
{
if (firstPageVotes == -1)
{
System.out.println("There was a problem on getting Topzone votes from server with rank "+firstPageRankNeeded+".");
}
if (currentVotes == -1)
{
System.out.println("There was a problem on getting Topzone server votes.");
}
return;
}
if (lastVotes == 0)
{
lastVotes = currentVotes;
Announcement.announceToAll("Topzone: Current vote count is "+currentVotes+".");
Announcement.announceToAll("Topzone: We need "+((lastVotes+voteRewardVotesDifference)-currentVotes)+" vote(s) for reward.");
if (Config.ALLOW_TOPZONE_GAME_SERVER_REPORT)
{
System.out.println("Server votes on topzone: "+currentVotes);
System.out.println("Votes needed for reward: "+((lastVotes+voteRewardVotesDifference)-currentVotes));
}
if (firstPageVotes-lastVotes <= 0)
{
Announcement.announceToAll("Topzone: We are in the top "+firstPageRankNeeded+" of topzone, so the reward will be big.");
if (Config.ALLOW_TOPZONE_GAME_SERVER_REPORT)
{
System.out.println("Server is on the top "+firstPageRankNeeded+" of topzone.");
}
}
else
{
Announcement.announceToAll("Topzone: We need "+(firstPageVotes-lastVotes)+" vote(s) to get to the top "+firstPageRankNeeded+" of topzone for big reward.");
if (Config.ALLOW_TOPZONE_GAME_SERVER_REPORT)
{
System.out.println("Server votes needed for top "+firstPageRankNeeded+": "+(firstPageVotes-lastVotes));
}
}
return;
}
if (currentVotes >= lastVotes+voteRewardVotesDifference)
{
Collection<L2PcInstance> pls = L2World.getInstance().getAllPlayers().values();
if (firstPageVotes-currentVotes <= 0)
{
if (Config.ALLOW_TOPZONE_GAME_SERVER_REPORT)
{
System.out.println("Server votes on topzone: "+currentVotes);
System.out.println("Server is on the top "+firstPageRankNeeded+" of topzone.");
System.out.println("Votes needed for next reward: "+((currentVotes+voteRewardVotesDifference)-currentVotes));
}
Announcement.announceToAll("Topzone: Everyone has been rewarded with big reward.");
Announcement.announceToAll("Topzone: Current vote count is "+currentVotes+".");
for (L2PcInstance p : pls)
{
boolean canReward = false;
String pIp = p.getClient().getConnection().getInetAddress().getHostAddress();
if (playerIps.containsKey(pIp))
{
int count = playerIps.get(pIp);
if (count < Config.TOPZONE_DUALBOXES_ALLOWED)
{
playerIps.remove(pIp);
playerIps.put(pIp, count+1);
canReward = true;
}
}
else
{
canReward = true;
playerIps.put(pIp, 1);
}
if (canReward)
{
for (int i = 0; i < Config.TOPZONE_BIG_REWARD.length; i++)
{
p.addItem("Vote reward.", Config.TOPZONE_BIG_REWARD[0], Config.TOPZONE_BIG_REWARD[1], p, true);
}
}
else
{
p.sendMessage("Already "+Config.TOPZONE_DUALBOXES_ALLOWED+" character(s) of your ip have been rewarded, so this character won't be rewarded.");
}
}
playerIps.clear();
}
else
{
if (Config.ALLOW_TOPZONE_GAME_SERVER_REPORT)
{
System.out.println("Server votes on topzone: "+currentVotes);
System.out.println("Server votes needed for top "+firstPageRankNeeded+": "+(firstPageVotes-lastVotes));
System.out.println("Votes needed for next reward: "+((currentVotes+voteRewardVotesDifference)-currentVotes));
}
Announcement.announceToAll("Topzone: Everyone has been rewarded with small reward.");
Announcement.announceToAll("Topzone: Current vote count is "+currentVotes+".");
Announcement.announceToAll("Topzone: We need "+(firstPageVotes-currentVotes)+" vote(s) to get to the top "+firstPageRankNeeded+" of topzone for big reward.");
for (L2PcInstance p : pls)
{
boolean canReward = false;
String pIp = p.getClient().getConnection().getInetAddress().getHostAddress();
if (playerIps.containsKey(pIp))
{
int count = playerIps.get(pIp);
if (count < Config.TOPZONE_DUALBOXES_ALLOWED)
{
playerIps.remove(pIp);
playerIps.put(pIp, count+1);
canReward = true;
}
}
else
{
canReward = true;
playerIps.put(pIp, 1);
}
if (canReward)
{
for (int i = 0; i < Config.TOPZONE_SMALL_REWARD.length; i++)
{
p.addItem("Vote reward.", Config.TOPZONE_SMALL_REWARD[0], Config.TOPZONE_SMALL_REWARD[1], p, true);
}
}
else
{
p.sendMessage("Already "+Config.TOPZONE_DUALBOXES_ALLOWED+" character(s) of your ip have been rewarded, so this character won't be rewarded.");
}
}
playerIps.clear();
}
lastVotes = currentVotes;
}
else
{
if (firstPageVotes-currentVotes <= 0)
{
if (Config.ALLOW_TOPZONE_GAME_SERVER_REPORT)
{
System.out.println("Server votes on topzone: "+currentVotes);
System.out.println("Server is on the top "+firstPageRankNeeded+" of topzone.");
System.out.println("Votes needed for next reward: "+((lastVotes+voteRewardVotesDifference)-currentVotes));
}
Announcement.announceToAll("Topzone: Current vote count is "+currentVotes+".");
Announcement.announceToAll("Topzone: We need "+((lastVotes+voteRewardVotesDifference)-currentVotes)+" vote(s) for big reward.");
}
else
{
if (Config.ALLOW_TOPZONE_GAME_SERVER_REPORT)
{
System.out.println("Server votes on topzone: "+currentVotes);
System.out.println("Server votes needed for top "+firstPageRankNeeded+": "+(firstPageVotes-lastVotes));
System.out.println("Votes needed for next reward: "+((lastVotes+voteRewardVotesDifference)-currentVotes));
}
Announcement.announceToAll("Topzone: Current vote count is "+currentVotes+".");
Announcement.announceToAll("Topzone: We need "+((lastVotes+voteRewardVotesDifference)-currentVotes)+" vote(s) for small reward.");
Announcement.announceToAll("Topzone: We need "+(firstPageVotes-currentVotes)+" vote(s) to get to the top "+firstPageRankNeeded+" of topzone for big reward.");
}
}
}
private static int getFirstPageRankVotes()
{
InputStreamReader isr = null;
BufferedReader br = null;
try
{
URLConnection con = new URL(page1Url).openConnection();
con.addRequestProperty("User-Agent", "Mozilla/4.76");
isr = new InputStreamReader(con.getInputStream());
br = new BufferedReader(isr);
String line;
while ((line = br.readLine()) != null)
{
if (line.contains("<div class=\"slr\">"+firstPageRankNeeded+"<div class=\"votes\">Votes:<br><span>"))
{
int votes = Integer.valueOf(line.split("<div class=\"slr\">"+firstPageRankNeeded+"<div class=\"votes\">Votes:<br><span>")[1].replace("</span></div></div>", ""));
return votes;
}
}
br.close();
isr.close();
}
catch (Exception e)
{
System.out.println(e);
System.out.println("Error while getting Hopzone server vote count.");
}
return -1;
}
private static int getVotes()
{
InputStreamReader isr = null;
BufferedReader br = null;
try
{
URLConnection con = new URL(topzoneUrl).openConnection();
con.addRequestProperty("User-Agent", "Mozilla/4.76");
isr = new InputStreamReader(con.getInputStream());
br = new BufferedReader(isr);
boolean got = false;
String line;
while ((line = br.readLine()) != null)
{
if (line.contains("<div class=\"rank\"><div class=\"votes2\">Votes:<br>") && !got)
{
got = true;
int votes = Integer.valueOf(line.split("<div class=\"rank\"><div class=\"votes2\">Votes:<br>")[1].replace("</div></div>", ""));
return votes;
}
}
br.close();
isr.close();
}
catch (Exception e)
{
System.out.println(e);
System.out.println("Error while getting server vote count.");
}
return -1;
}
}