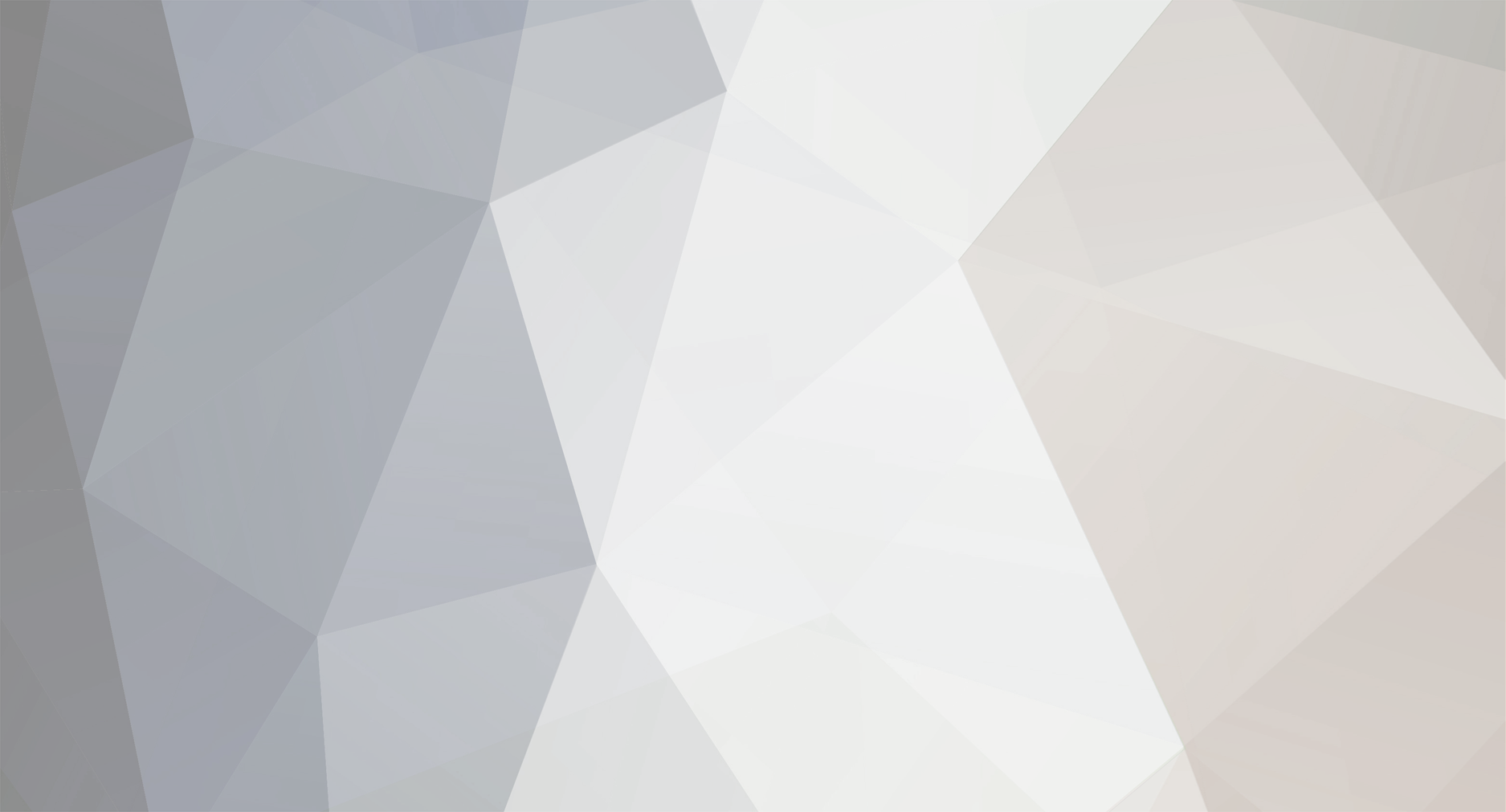
dimityr203
-
Posts
12 -
Credits
0 -
Joined
-
Last visited
-
Feedback
0%
Content Type
Articles
Profiles
Forums
Store
Posts posted by dimityr203
-
-
Hello here is for Acis.
Index: config/players.properties =================================================================== --- config/players.properties (revision 6) +++ config/players.properties (working copy) @@ -294,4 +288,55 @@ MaxBuffsAmount = 20 # Store buffs/debuffs on user logout? -StoreSkillCooltime = True \ No newline at end of file +StoreSkillCooltime = True + +# ---------------------- +# Enchant Announce - +# ---------------------- +# Announce when a player successfully enchant an item to x +# Default: False +EnableEnchantAnnounce = False +# The value of x is... set it here (No have default value) +EnchantAnnounceLevel = 6 \ No newline at end of file Index: java/net/sf/l2j/Config.java =================================================================== --- java/net/sf/l2j/Config.java (revision 6) +++ java/net/sf/l2j/Config.java (working copy) @@ -495,6 +492,25 @@ public static boolean STORE_SKILL_COOLTIME; public static int BUFFS_MAX_AMOUNT; + public static boolean ENABLE_ENCHANT_ANNOUNCE; + public static int ENCHANT_ANNOUNCE_LEVEL; + // -------------------------------------------------- // Server // -------------------------------------------------- @@ -1204,6 +1228,48 @@ BUFFS_MAX_AMOUNT = players.getProperty("MaxBuffsAmount", 20); STORE_SKILL_COOLTIME = players.getProperty("StoreSkillCooltime", true); + + ENABLE_ENCHANT_ANNOUNCE = players.getProperty("EnableEnchantAnnounce", false); + ENCHANT_ANNOUNCE_LEVEL = players.getProperty("EnchantAnnounceLevel", 16); } /** Index: java/net/sf/l2j/gameserver/network/clientpackets/RequestEnchantItem.java =================================================================== --- java/net/sf/l2j/gameserver/network/clientpackets/RequestEnchantItem.java (revision 6) +++ java/net/sf/l2j/gameserver/network/clientpackets/RequestEnchantItem.java (working copy) @@ -34,6 +34,7 @@ import net.sf.l2j.gameserver.network.serverpackets.ItemList; import net.sf.l2j.gameserver.network.serverpackets.StatusUpdate; import net.sf.l2j.gameserver.network.serverpackets.SystemMessage; +import net.sf.l2j.gameserver.util.Broadcast; import net.sf.l2j.gameserver.util.Util; public final class RequestEnchantItem extends AbstractEnchantPacket @@ -129,11 +130,15 @@ // announce the success SystemMessage sm; + int nextEnchantLevel = item.getEnchantLevel() + 1; + if (item.getEnchantLevel() == 0) { sm = SystemMessage.getSystemMessage(SystemMessageId.S1_SUCCESSFULLY_ENCHANTED); sm.addItemName(item.getItemId()); activeChar.sendPacket(sm); + if(Config.ENABLE_ENCHANT_ANNOUNCE && Config.ENCHANT_ANNOUNCE_LEVEL == 0) + Broadcast.announceToOnlinePlayers("Congratulations to " + activeChar.getName() + "! Your " + item.getItemName() + " has been successfully enchanted to +" + nextEnchantLevel); } else { @@ -141,6 +146,8 @@ sm.addNumber(item.getEnchantLevel()); sm.addItemName(item.getItemId()); activeChar.sendPacket(sm); + if(Config.ENABLE_ENCHANT_ANNOUNCE && Config.ENCHANT_ANNOUNCE_LEVEL <= item.getEnchantLevel()) + Broadcast.announceToOnlinePlayers("Congratulations to " + activeChar.getName() + "! Your " + item.getItemName() + " has been successfully enchanted to +" + nextEnchantLevel); } item.setEnchantLevel(item.getEnchantLevel() + 1);
-
I downloaded it, the problem is fixed when i renamed system1 to system, i logged in and i find an empty server, i dont wanna think how many people u lost from these stupid bugs... anyway, good luck with this.
Oki thx :)
-
It doesnt working for me, when i press on how to connect tab i get an error which says: There's nothing here, yet. Start your website.
Use save as.
-
Dude Hot to connect tab is broken, where do i dl patch from??? :okey:
Its working i downloaded just now
-
Try download patch again. I use new one.
-
Oki i make a new patch
-
Well, I downloaded the client linked from your website. Still not working.
Unrar patch in game directory, lunch l2.exe from system1 folder?
-
Client doesn't respond when I try to create a new character and click on the race arrow. That's quite bizarre, never happened to me before. Tried it 2-3 times.
May be old interlude client.
-
Armors and weapons in style epic and some coins: for nobless, for hero....
-
Server Open: 15.04.2017.Server Rates:- Adena: 5 000x.- XP: 5 000x.- SP: 5 000x.- Drop: 1x.- PartyXp: 1x.- PartySp: 1x.- Starting character: 40 LvL.Enchant rates:- Safe enchant +4.- Blessed scrolls max enchant (+16).- Crystal and simple scrolls max enchant (+20).- Simple enchant scrolls chance - 70%.- Blessed enchant scrolls chance - 100%.- Crystal enchant scrolls chance - 100%.Unique features:Custom character starting points.Gm ShopGlobal GKVote ManagerNPC BufferMax count of buffs - 76.Npc skill enchanter.Casino NPCNo grade limit.No weight limit.Main towns - Giran.Working all castle sieges.Wedding system.Unique farming areas.Max subclasses - 5.Free and no quest class change.Free and no quest sub class.Pvp/pk show on title.Online password change.Unique monsters.Interlude retail skills.Server up-time [24/7] [99]%.Custom items.Voiced commands:.tvtjoin .tvtleave - Join or leave tvt event..ctfjoin .ctfleave - Join or leave ctf event..dmjoin .dmleave - Join of leave dm event..menuEvent system:- TVT event [12:00 17:00 20:00 23:00] hours.- CTF event [15:00 18:00 21:00] hours.- DM event [11:00 14:00 18:00 22:10] hours.- Top PvP and PK player event every 24H.- Town war event every day.- Casino event working [24/7].- Unique event shop.Olympiad game:- Retail olympiad game.- Competition period [1] week.- Olympiad start time [18:00] end [00:00].- Olympoiad start/end times can be found in olympiad manager.- Friendly GM's- Try this server for fun!Web Site: http://l2hunters.net
-
ItemSkill class ---->> ItemSkills is = new ItemSkills();
Any ideas how to fix it?? Frozen rev 1132
Potions msu = new Potions();msu.usePotion(activeChar, 2005, 1);
Noblesse Killing Barakiel L2Jacis
in Server Shares & Files [L2J]
Posted
For rev. 393